-
Notifications
You must be signed in to change notification settings - Fork 2
tutorial.basic.10
Last time we looked at the grid structure, now we are going to look at framesets! If you have used something HTML before, framesets should be very familiar to you. Anyways for those that don't know, letβs get down what a frameset exactly is. A frameset is an flexible structure. With grids all of your cells are bound to each other: you can only resize single rows or columns. This is not the case for framesets. With framesets you can create stuff like this:

You should see that it is not practical to make this example using grids. However, this example is super useful. On the top frame you could put buttons for example, and on the left side you could put a menu. Then in the middle you could have it display something else.
Now letβs get start understanding how we make these slick structures. When working with framesets, you usually take all of these steps:
- Create the frameset using uiz_frameset_create. (uiz_c(obj_uiZ_frameset) will work just as well).
- Make some amount of divisions or cuts using the uiz_framedivision_ scripts.
- Fixing the frameset using uiz_fix().
The first and last part should be pretty straight forward right now. The second thing you need to do is a little harder though, but itβs the step that will define what your frameset looks like.
Defining your frameset exists out of 2 different things: horizontal and vertical divisions. When doing a horizontal division, just think of a horizontal line (or multiple lines) cutting a frame into pieces. When doing a vertical division, do the same with vertical lines.
If you look at image 49, you can see that it exists out of 2 divisions, one horizontal and one vertical. If you look closely, you can also see some structure in it: The horizontal division has been done first, after which a second vertical division has been made inside the bottom cell of the horizontal division.

Now letβs look at some functions. The function for a horizontal cut is uiz_frameset_divide_objects_horizontal, and the one for a vertical cut is: uiz_frameset_divide_objects_vertical. Now would be a great time to also look at the frameset page. Come on, find the division functions. These functions return a frameset anchor, which weβll learn about later. These functions both take at least, but are not limited to 5 arguments. The first argument can refer to a few different objects: The frameset or a frame inside the frameset. What it can NEVER refer to are: frame anchors (what a uiz_frameset_divide_objects_ returns) or a frameset that already has a division.
Let's see these functions in action in order to create a simple horizontal division. Again, we are using gradient squares to make things easily visible
EXAMPLE 37:
//init uiz
uiz_init()
//create frameset
frset = uiz_frameset_create()
//divide horizontally
div1 = uiz_frameset_divide_objects_horizontal(frset, 1,dp,obj_uiZ_gradientsquare, 1,xtra,obj_uiZ_gradientsquare);
//fix frameset
uiz_fix(frset)
This yields:

Note that with uiz_frameset_divide_objects_ any amount of divisions can be made within a single function, just just have to add an extra set of arguments. (Like 50,px,obj_uiZ_rotator for example).
Also, youβre not done with your divisions by just calling a uiz_frameset_divide_objects_ script. You need to βgetβ the newly created frames. With βgettingβ I mean getting an instance id of a frame. For this youβll need a frame anchor. Frame anchors act like in-betweens, an object which stitches the frames in your frameset together. It is not that important to have a very deep understanding of frame anchors, but they are needed for getting the frames in framesets. To do this, use the uiz_frameset_getObject function. (Look it up in the manual!) You see that the second argument is called "position". When making a horizontal division, the top most frame that is created is at "position" 0, while the second from top frame is at "1", etc.. When making vertical divisions, the same principle applies but then from left to right.
Once you have gotten your objects in your frameset, you can use those objects to make more subdivisions like this:
EXAMPLE 37:
//init uiz
uiz_init()
//create frameset
frset = uiz_frameset_create()
//divide horizontally
div1 = uiz_frameset_divide_objects_horizontal(frset, 1,dp,obj_uiZ_gradientsquare, 1,xtra,obj_uiZ_gradientsquare);
//divide vertically
bottomFrame = uiz_frameset_getObject(div1, 1);//get the second (bottom) frame of the first division we made
div2 = uiz_frameset_divide_objects_vertical(bottomFrame, 0.1,fc,obj_uiZ_gradientsquare, 1,xtra,obj_uiZ_gradientsquare);
//fix
uiz_fix(frset);
//get all frames for later reference:
leftFrame = uiz_frameset_getObject(div2, 0);
mainFrame = uiz_frameset_getObject(div2, 1);
topBar = uiz_frameset_getObject(div1, 0);

Now, the top frame is stored in a variable called topBar the left bar is stored in a variable called leftFrame and the right frame is stored in a variable called mainFrame. βdiv1β and βdiv2β refer to frame anchors in this code. You should NOT call uiz_frameset_divide_objects_ on "frset" anymore, since this will result in a crash.
As youβve seen in our examples, we can use βsizetypesβ on framesets. Inside our uiz_frameset_divide_objects_ script, we can give it βpxβ, βdpβ, βfcβ, and βxtraβ, all of these work like explained in previous tutorials. Whatβs also nice about the uiz_frameset_divide_ functions, is that they support more than 2 frames. You might want to create a row of 3 frames, or 5 frames. The division functions supports as many frames as the amount of arguments game maker can handle for scripts. Just make sure that you specify both a size, and a size type for every frame (in the case of the _objects variant like we used above you also need to specify an object type). Here is an example, where weβre getting more frames from one division:
EXAMPLE 39:
//init uiz
uiz_init()
//create frameset
frset = uiz_frameset_create()
//divide horizontally
div1 = uiz_frameset_divide_objects_horizontal(frset, 1,dp,obj_uiZ_gradientsquare, 1,xtra,obj_uiZ_gradientsquare, 50,px,obj_uiZ_button);
//divide vertically
bottomFrame = uiz_frameset_getObject(div1, 1);//get the second (bottom) frame of the first division we made
div2 = uiz_frameset_divide_objects_vertical(bottomFrame, 0.1,fc,obj_uiZ_gradientsquare, 1,xtra,obj_uiZ_gradientsquare, 3,xtra,obj_uiZ_rotator, 30,px,obj_uiZ_gradientsquare,);
uiz_fix(frset)
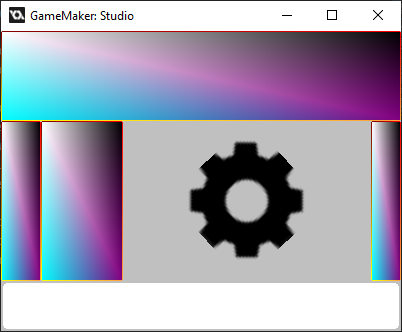
Now you should fully understand how to use framesets. There are a few more functions about which you can find more information in the manual. Those functions arenβt that important and you wonβt be using them that often. You should by now be able to make structured ui.
In this tutorial you learned:
- How to create framesets
- How to make divisions in framesets
- How to make divisions in framesets which results in more than 2 frames
- How to fill up a frameset with objects
Next time weβll talk about windows, another important feature of uiz.
πTutorials
Basics 1: Basic positioning
Basics 2: Parenting system
Basics 3: Advanced positioning
Basics 4: Advanced sizing and set point
Basics 5: Canvas and containment
Basics 6: Alpha and depth
Basics 7: Using the manual and Animations
Basics 8: Object backgrounds
Basics 9: Grids
Basics 10: Framesets
Basics 11: Windows
Basics 12: Scroll bars
βοΈ Positioning
π Depth
π Structures
π Objects
obj_uiZ_3waybutton
obj_uiZ_button
obj_uiZ_checkbox
obj_uiZ_clock
obj_uiZ_colorbox
obj_uiZ_cover
obj_uiZ_drawdslist
obj_uiZ_dropdown
obj_uiZ_easybutton
obj_uiZ_frame
obj_uiZ_framescrollbar
obj_uiZ_functionbar
obj_uiZ_gradientsquare
obj_uiZ_gradientroundrect
obj_uiZ_gridlist
obj_uiZ_huesquare
obj_uiZ_loadingbar
obj_uiZ_loadingcircle
obj_uiZ_menubutton
obj_uiZ_mousemenu
obj_uiZ_radiobox
obj_uiZ_rotator
obj_uiZ_slider
obj_uiZ_scrollbar
obj_uiZ_slider_2col
obj_uiZ_slickslider
obj_uiZ_slideframe
obj_uiZ_sprbutton
obj_uiZ_spriteanimationbutton
obj_uiZ_spritecounter
obj_uiZ_stringbox
obj_uiZ_sliderstruct
obj_uiZ_surfacecanvas
obj_uiZ_sprite
obj_uiZ_square
obj_uiZ_squarebutton
obj_uiZ_swipicon
obj_uiZ_switch
obj_uiZ_tabslider
obj_uiZ_tabs
obj_uiZ_treelist
obj_uiZ_text
obj_uiZ_text_background
obj_uiZ_textarea
obj_uiZ_valuebox
π Strings
uiz_addChar
uiz_changechar
uiz_charCanHaveAddon
uiz_returnCharAddon
uiz_charIsNumber
uiz_charIsNumberOrText
uiz_getlines
uiz_gettext_contained
uiz_gettextlines_contained
uiz_getValidVariableName
uiz_isSpaceChar
uiz_lastStringChars
uiz_removeChar
uiz_replaceChars_
uiz_string_copy
uiz_string_digits
uiz_string_format
uiz_string_fromReal
uiz_string_real_getFracLength
uiz_string_real_getIntLength
uiz_string_repeat
uiz_string_replace
uiz_string_pos_at
uiz_stringUntilNewline