diff --git a/README.md b/README.md
index 5d336cf..bac925b 100644
--- a/README.md
+++ b/README.md
@@ -11,16 +11,16 @@
-Bagel is a little native iOS network debugger. It's not a proxy debugger so you don't have to mess around with certificates, proxy settings etc. As long as your iOS devices and your Mac are in the same network, you can view the network traffic of your apps seperated by the devices or simulators.
+Bagel is a little native iOS/Android network debugger. It's not a proxy debugger so you don't have to mess around with certificates, proxy settings etc. As long as your iOS/Android devices and your Mac are in the same network, you can view the network traffic of your apps seperated by the devices or simulators.
## Preview
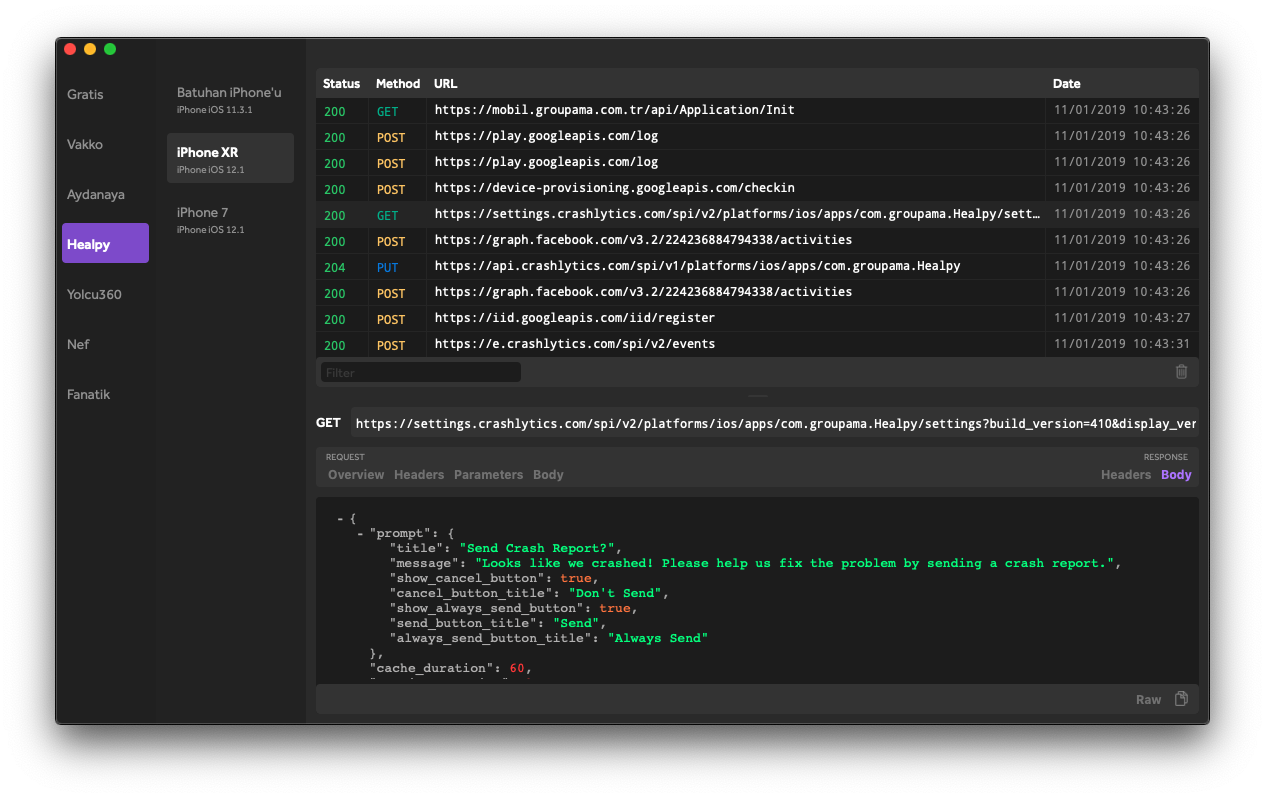
-## Installation
-#### Install Mac App
+## Install Mac App
- Clone the repo.
- Install pods.
- Build and archive the project.
-#### Install iOS Client
+
+## Install iOS Client
#### CocoaPods
```shhttps://img.shields.io/badge/version-1.3.1-blue.svg?style=flat
pod 'Bagel', '~> 1.4.0'
@@ -71,7 +71,72 @@ bagelConfig.netserviceName = ""
Bagel.start(bagelConfig)
```
-If you change Netservice parameters in your app, you should also change them on desktop client.
+
+## Install Android client
+#### Dependency
+* Add the below dependency in your preferred build system
+ ```groovy
+ implementation 'com.simform:bagel:1.0.1'
+ ```
+
+### Usage
+In order to start Bagel we need to start the client and add a interceptor to intercept [OkHttp](https://square.github.io/okhttp/) API calls.
+
+* You can start client in any one of the way you like from below
+ 1. Start client when `Application` class starts
+ ```kotlin
+ class App : Application() {
+ override fun onCreate() {
+ super.onCreate()
+ if (BuildConfig.DEBUG) { // Only expose in debug
+ Bagel.start(context)
+ }
+ }
+ }
+ ```
+ 2. Start client using [AppStartup](https://developer.android.com/topic/libraries/app-startup)
+ ```kotlin
+ class BagelInitializer : Initializer {
+ override fun create(context: Context) {
+ if (BuildConfig.DEBUG) { // Only expose in debug
+ Bagel.start(context)
+ }
+ }
+
+ override fun dependencies(): MutableList>> =
+ mutableListOf()
+ }
+ ```
+
+* Add [OkHttp](https://square.github.io/okhttp/) interceptor (**This is required to route API call details**)
+ * While building your `OkHttp` client create interceptor instance as below
+ ```kotlin
+ OkHttpClient.Builder()
+ .apply {
+ if (BuildConfig.DEBUG) { // Only expose in debug
+ val bagelInterceptor = BagelInterceptor.getInstance()
+ addInterceptor(bagelInterceptor)
+ }
+ }
+ . // Add all other interceptor and configurations
+ .build()
+ ```
+
+### Configuring Bagel
+By default, Bagel gets your project name and device information. Desktop client uses these informations to separate projects and devices. You can configure `projectName` and `netServiceType` if you wish:
+
+```kotlin
+val bagelConfiguration = BagelConfiguration
+ .getDefault(context)
+ .copy(projectName = "Bagel")
+
+Bagel.start(
+ context,
+ bagelConfiguration
+)
+```
+
+#### Note : If you change `netServiceType` parameter in your app, you should also change them on desktop client.
License
----
diff --git a/android/.gitignore b/android/.gitignore
new file mode 100644
index 0000000..c8ee023
--- /dev/null
+++ b/android/.gitignore
@@ -0,0 +1,57 @@
+# Built application files
+*.apk
+*.ap_
+
+# Files for the ART/Dalvik VM
+*.dex
+
+# Java class files
+*.class
+
+# Generated files
+bin/
+gen/
+out/
+
+# Gradle files
+/.idea
+.gradle/
+build/
+
+# Local configuration file (sdk path, etc)
+local.properties
+
+# Proguard folder generated by Eclipse
+proguard/
+
+# Log Files
+*.log
+
+# Android Studio Navigation editor temp files
+.navigation/
+
+# Android Studio captures folder
+captures/
+
+# Intellij
+*.iml
+.idea/workspace.xml
+.idea/tasks.xml
+.idea/gradle.xml
+.idea/dictionaries
+.idea/libraries
+app/.idea/
+
+# Mac
+*.DS_Store
+
+# Keystore files
+*.jks
+
+# External native build folder generated in Android Studio 2.2 and later
+.externalNativeBuild
+
+# Temporary API docs
+docs/api
+
+artifacts
\ No newline at end of file
diff --git a/android/app/.gitignore b/android/app/.gitignore
new file mode 100644
index 0000000..d849f4f
--- /dev/null
+++ b/android/app/.gitignore
@@ -0,0 +1,55 @@
+# Built application files
+*.apk
+*.ap_
+
+# Files for the ART/Dalvik VM
+*.dex
+
+# Java class files
+*.class
+
+# Generated files
+bin/
+gen/
+out/
+
+# Gradle files
+/.idea
+.gradle/
+build/
+
+# Local configuration file (sdk path, etc)
+local.properties
+
+# Proguard folder generated by Eclipse
+proguard/
+
+# Log Files
+*.log
+
+# Android Studio Navigation editor temp files
+.navigation/
+
+# Android Studio captures folder
+captures/
+
+# Intellij
+*.iml
+.idea/workspace.xml
+.idea/tasks.xml
+.idea/gradle.xml
+.idea/dictionaries
+.idea/libraries
+app/.idea/
+
+# Mac
+*.DS_Store
+
+# Keystore files
+*.jks
+
+# External native build folder generated in Android Studio 2.2 and later
+.externalNativeBuild
+
+# Temporary API docs
+docs/api
diff --git a/android/app/build.gradle.kts b/android/app/build.gradle.kts
new file mode 100644
index 0000000..a9ec7ba
--- /dev/null
+++ b/android/app/build.gradle.kts
@@ -0,0 +1,79 @@
+plugins {
+ alias(libs.plugins.android.application)
+ alias(libs.plugins.jetbrains.kotlin.android)
+ alias(libs.plugins.compose.compiler)
+ alias(libs.plugins.kotlinx.serialization)
+ alias(libs.plugins.hilt)
+ alias(libs.plugins.ksp)
+}
+
+android {
+ namespace = "com.simformsolutions.bagelandroid"
+ compileSdk = libs.versions.compileSdk.get().toInt()
+
+ defaultConfig {
+ applicationId = "com.simformsolutions.bagelandroid"
+ minSdk = libs.versions.minSdk.get().toInt()
+ targetSdk = libs.versions.targetSdk.get().toInt()
+ versionCode = 1
+ versionName = "1.0"
+
+ testInstrumentationRunner = "androidx.test.runner.AndroidJUnitRunner"
+ vectorDrawables {
+ useSupportLibrary = true
+ }
+ }
+
+ buildTypes {
+ release {
+ isMinifyEnabled = true
+ proguardFiles(
+ getDefaultProguardFile("proguard-android-optimize.txt"),
+ "proguard-rules.pro"
+ )
+ }
+ }
+ compileOptions {
+ sourceCompatibility = JavaVersion.VERSION_1_8
+ targetCompatibility = JavaVersion.VERSION_1_8
+ }
+ kotlinOptions {
+ jvmTarget = "1.8"
+ }
+ buildFeatures {
+ compose = true
+ }
+}
+
+dependencies {
+ // Uncomment this line to check locally published version
+// implementation("com.simform:bagel:1.0.1")
+ // Comment this line to check locally published version
+ implementation(project(":bagel"))
+ implementation(libs.androidx.core.ktx)
+ implementation(libs.androidx.lifecycle.runtime.ktx)
+ implementation(libs.androidx.activity.compose)
+ implementation(platform(libs.androidx.compose.bom))
+ implementation(libs.androidx.ui)
+ implementation(libs.androidx.ui.graphics)
+ implementation(libs.androidx.ui.tooling.preview)
+ implementation(libs.androidx.material3)
+ implementation(libs.timber)
+ implementation(libs.androidx.lifecycle.runtime.compose)
+ implementation(libs.kotlinx.serialization.json)
+ implementation(libs.androidx.startup.runtime)
+ implementation(platform(libs.retrofit.bom))
+ implementation(libs.retrofit)
+ implementation(libs.hilt.android)
+ implementation(libs.logging.interceptor)
+ implementation(libs.retrofit2.kotlinx.serialization.converter)
+ implementation(libs.androidx.hilt.navigation.compose)
+ ksp(libs.hilt.android.compiler)
+ testImplementation(libs.junit)
+ androidTestImplementation(libs.androidx.junit)
+ androidTestImplementation(libs.androidx.espresso.core)
+ androidTestImplementation(platform(libs.androidx.compose.bom))
+ androidTestImplementation(libs.androidx.ui.test.junit4)
+ debugImplementation(libs.androidx.ui.tooling)
+ debugImplementation(libs.androidx.ui.test.manifest)
+}
\ No newline at end of file
diff --git a/android/app/proguard-rules.pro b/android/app/proguard-rules.pro
new file mode 100644
index 0000000..481bb43
--- /dev/null
+++ b/android/app/proguard-rules.pro
@@ -0,0 +1,21 @@
+# Add project specific ProGuard rules here.
+# You can control the set of applied configuration files using the
+# proguardFiles setting in build.gradle.
+#
+# For more details, see
+# http://developer.android.com/guide/developing/tools/proguard.html
+
+# If your project uses WebView with JS, uncomment the following
+# and specify the fully qualified class name to the JavaScript interface
+# class:
+#-keepclassmembers class fqcn.of.javascript.interface.for.webview {
+# public *;
+#}
+
+# Uncomment this to preserve the line number information for
+# debugging stack traces.
+#-keepattributes SourceFile,LineNumberTable
+
+# If you keep the line number information, uncomment this to
+# hide the original source file name.
+#-renamesourcefileattribute SourceFile
\ No newline at end of file
diff --git a/android/app/src/androidTest/java/com/simformsolutions/bagelandroid/ExampleInstrumentedTest.kt b/android/app/src/androidTest/java/com/simformsolutions/bagelandroid/ExampleInstrumentedTest.kt
new file mode 100644
index 0000000..640006e
--- /dev/null
+++ b/android/app/src/androidTest/java/com/simformsolutions/bagelandroid/ExampleInstrumentedTest.kt
@@ -0,0 +1,24 @@
+package com.simformsolutions.bagelandroid
+
+import androidx.test.platform.app.InstrumentationRegistry
+import androidx.test.ext.junit.runners.AndroidJUnit4
+
+import org.junit.Test
+import org.junit.runner.RunWith
+
+import org.junit.Assert.*
+
+/**
+ * Instrumented test, which will execute on an Android device.
+ *
+ * See [testing documentation](http://d.android.com/tools/testing).
+ */
+@RunWith(AndroidJUnit4::class)
+class ExampleInstrumentedTest {
+ @Test
+ fun useAppContext() {
+ // Context of the app under test.
+ val appContext = InstrumentationRegistry.getInstrumentation().targetContext
+ assertEquals("com.simformsolutions.bagelandroid", appContext.packageName)
+ }
+}
\ No newline at end of file
diff --git a/android/app/src/main/AndroidManifest.xml b/android/app/src/main/AndroidManifest.xml
new file mode 100644
index 0000000..cab5f88
--- /dev/null
+++ b/android/app/src/main/AndroidManifest.xml
@@ -0,0 +1,46 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
\ No newline at end of file
diff --git a/android/app/src/main/java/com/simformsolutions/bagelandroid/BagelApp.kt b/android/app/src/main/java/com/simformsolutions/bagelandroid/BagelApp.kt
new file mode 100644
index 0000000..cd1b00a
--- /dev/null
+++ b/android/app/src/main/java/com/simformsolutions/bagelandroid/BagelApp.kt
@@ -0,0 +1,7 @@
+package com.simformsolutions.bagelandroid
+
+import android.app.Application
+import dagger.hilt.android.HiltAndroidApp
+
+@HiltAndroidApp
+class BagelApp : Application()
diff --git a/android/app/src/main/java/com/simformsolutions/bagelandroid/di/NetworkModule.kt b/android/app/src/main/java/com/simformsolutions/bagelandroid/di/NetworkModule.kt
new file mode 100644
index 0000000..6464136
--- /dev/null
+++ b/android/app/src/main/java/com/simformsolutions/bagelandroid/di/NetworkModule.kt
@@ -0,0 +1,75 @@
+package com.simformsolutions.bagelandroid.di
+
+import com.jakewharton.retrofit2.converter.kotlinx.serialization.asConverterFactory
+import com.simform.bagel.intercept.BagelInterceptor
+import com.simformsolutions.bagelandroid.remote.NetworkService
+import dagger.Module
+import dagger.Provides
+import dagger.hilt.InstallIn
+import dagger.hilt.components.SingletonComponent
+import kotlinx.serialization.ExperimentalSerializationApi
+import kotlinx.serialization.json.Json
+import okhttp3.MediaType.Companion.toMediaType
+import okhttp3.OkHttpClient
+import okhttp3.logging.HttpLoggingInterceptor
+import retrofit2.Retrofit
+import retrofit2.create
+import java.util.concurrent.TimeUnit
+import javax.inject.Singleton
+
+private const val CONNECT_TIMEOUT_SECONDS = 10L
+private const val READ_TIMEOUT_SECONDS = 5L
+private const val WRITE_TIMEOUT_SECONDS = 5L
+
+@Module
+@InstallIn(SingletonComponent::class)
+object NetworkModule {
+
+ @OptIn(ExperimentalSerializationApi::class)
+ @Provides
+ @Singleton
+ fun providesNetworkJson(): Json = Json {
+ ignoreUnknownKeys = true
+ explicitNulls = false
+ coerceInputValues = false
+ prettyPrint = true
+ encodeDefaults = true
+ }
+
+ @Provides
+ @Singleton
+ fun provideLoggerInterceptor(): HttpLoggingInterceptor = HttpLoggingInterceptor().apply {
+ level = HttpLoggingInterceptor.Level.BODY
+ }
+
+ @Provides
+ @Singleton
+ fun provideBagelInterceptor(): BagelInterceptor =
+ BagelInterceptor.getInstance()
+
+ @Provides
+ @Singleton
+ fun provideApiClient(
+ logger: HttpLoggingInterceptor,
+ bagelInterceptor: BagelInterceptor
+ ): OkHttpClient =
+ OkHttpClient.Builder()
+ .addInterceptor(bagelInterceptor)
+ .addInterceptor(logger)
+ .connectTimeout(CONNECT_TIMEOUT_SECONDS, TimeUnit.SECONDS)
+ .readTimeout(READ_TIMEOUT_SECONDS, TimeUnit.SECONDS)
+ .writeTimeout(WRITE_TIMEOUT_SECONDS, TimeUnit.SECONDS)
+ .build()
+
+ @Provides
+ @Singleton
+ fun provideRetrofit(networkJson: Json, client: OkHttpClient): Retrofit = Retrofit.Builder()
+ .baseUrl("https://google.com")
+ .client(client)
+ .addConverterFactory(networkJson.asConverterFactory("application/json".toMediaType()))
+ .build()
+
+ @Provides
+ @Singleton
+ fun provideNetworkService(retrofit: Retrofit): NetworkService = retrofit.create()
+}
\ No newline at end of file
diff --git a/android/app/src/main/java/com/simformsolutions/bagelandroid/di/RepositoryModule.kt b/android/app/src/main/java/com/simformsolutions/bagelandroid/di/RepositoryModule.kt
new file mode 100644
index 0000000..c04bd92
--- /dev/null
+++ b/android/app/src/main/java/com/simformsolutions/bagelandroid/di/RepositoryModule.kt
@@ -0,0 +1,17 @@
+package com.simformsolutions.bagelandroid.di
+
+import com.simformsolutions.bagelandroid.domain.repository.NetworkRepository
+import com.simformsolutions.bagelandroid.domain.repository.NetworkRepositoryImpl
+import dagger.Binds
+import dagger.Module
+import dagger.hilt.InstallIn
+import dagger.hilt.components.SingletonComponent
+import javax.inject.Singleton
+
+@Module
+@InstallIn(SingletonComponent::class)
+interface RepositoryModule {
+ @Singleton
+ @Binds
+ fun bindNetworkRepository(impl: NetworkRepositoryImpl): NetworkRepository
+}
\ No newline at end of file
diff --git a/android/app/src/main/java/com/simformsolutions/bagelandroid/domain/repository/NetworkRepository.kt b/android/app/src/main/java/com/simformsolutions/bagelandroid/domain/repository/NetworkRepository.kt
new file mode 100644
index 0000000..67b7d66
--- /dev/null
+++ b/android/app/src/main/java/com/simformsolutions/bagelandroid/domain/repository/NetworkRepository.kt
@@ -0,0 +1,8 @@
+package com.simformsolutions.bagelandroid.domain.repository
+
+import retrofit2.Response
+
+interface NetworkRepository {
+
+ suspend fun getHotCoffee()
+}
\ No newline at end of file
diff --git a/android/app/src/main/java/com/simformsolutions/bagelandroid/domain/repository/NetworkRepositoryImpl.kt b/android/app/src/main/java/com/simformsolutions/bagelandroid/domain/repository/NetworkRepositoryImpl.kt
new file mode 100644
index 0000000..37bbe1e
--- /dev/null
+++ b/android/app/src/main/java/com/simformsolutions/bagelandroid/domain/repository/NetworkRepositoryImpl.kt
@@ -0,0 +1,19 @@
+package com.simformsolutions.bagelandroid.domain.repository
+
+import com.simformsolutions.bagelandroid.remote.NetworkService
+import kotlinx.coroutines.Dispatchers
+import kotlinx.coroutines.withContext
+import retrofit2.Response
+import javax.inject.Inject
+import javax.inject.Singleton
+
+@Singleton
+class NetworkRepositoryImpl @Inject constructor(
+ private val networkService: NetworkService
+) : NetworkRepository {
+ override suspend fun getHotCoffee() {
+ withContext(Dispatchers.IO) {
+ networkService.getHotCoffee()
+ }
+ }
+}
\ No newline at end of file
diff --git a/android/app/src/main/java/com/simformsolutions/bagelandroid/initializer/BagelInitializer.kt b/android/app/src/main/java/com/simformsolutions/bagelandroid/initializer/BagelInitializer.kt
new file mode 100644
index 0000000..36c8547
--- /dev/null
+++ b/android/app/src/main/java/com/simformsolutions/bagelandroid/initializer/BagelInitializer.kt
@@ -0,0 +1,15 @@
+package com.simformsolutions.bagelandroid.initializer
+
+import android.content.Context
+import androidx.startup.Initializer
+import com.simform.bagel.Bagel
+import com.simform.bagel.config.BagelConfiguration
+
+class BagelInitializer : Initializer {
+ override fun create(context: Context) {
+ Bagel.start(context, BagelConfiguration.getDefault(context).copy(projectName = "Bagel"))
+ }
+
+ override fun dependencies(): MutableList>> =
+ mutableListOf(TimberInitializer::class.java)
+}
\ No newline at end of file
diff --git a/android/app/src/main/java/com/simformsolutions/bagelandroid/initializer/TimberInitializer.kt b/android/app/src/main/java/com/simformsolutions/bagelandroid/initializer/TimberInitializer.kt
new file mode 100644
index 0000000..3952951
--- /dev/null
+++ b/android/app/src/main/java/com/simformsolutions/bagelandroid/initializer/TimberInitializer.kt
@@ -0,0 +1,14 @@
+package com.simformsolutions.bagelandroid.initializer
+
+import android.content.Context
+import androidx.startup.Initializer
+import timber.log.Timber
+
+class TimberInitializer : Initializer {
+ override fun create(context: Context) {
+ Timber.plant(Timber.DebugTree())
+ Timber.d("TimberInitializer is initialized")
+ }
+
+ override fun dependencies(): List>> = emptyList()
+}
\ No newline at end of file
diff --git a/android/app/src/main/java/com/simformsolutions/bagelandroid/remote/NetworkService.kt b/android/app/src/main/java/com/simformsolutions/bagelandroid/remote/NetworkService.kt
new file mode 100644
index 0000000..7c23129
--- /dev/null
+++ b/android/app/src/main/java/com/simformsolutions/bagelandroid/remote/NetworkService.kt
@@ -0,0 +1,10 @@
+package com.simformsolutions.bagelandroid.remote
+
+import retrofit2.Response
+import retrofit2.http.GET
+
+interface NetworkService {
+
+ @GET("https://api.sampleapis.com/coffee/hot")
+ suspend fun getHotCoffee(): Unit
+}
\ No newline at end of file
diff --git a/android/app/src/main/java/com/simformsolutions/bagelandroid/ui/MainActivity.kt b/android/app/src/main/java/com/simformsolutions/bagelandroid/ui/MainActivity.kt
new file mode 100644
index 0000000..ef0b3b4
--- /dev/null
+++ b/android/app/src/main/java/com/simformsolutions/bagelandroid/ui/MainActivity.kt
@@ -0,0 +1,46 @@
+package com.simformsolutions.bagelandroid.ui
+
+import android.os.Bundle
+import androidx.activity.ComponentActivity
+import androidx.activity.compose.setContent
+import androidx.activity.enableEdgeToEdge
+import androidx.compose.foundation.layout.fillMaxSize
+import androidx.compose.foundation.layout.padding
+import androidx.compose.material3.Scaffold
+import androidx.compose.runtime.Composable
+import androidx.compose.ui.Modifier
+import androidx.compose.ui.tooling.preview.Preview
+import com.simformsolutions.bagelandroid.ui.main.MainRoute
+import com.simformsolutions.bagelandroid.ui.main.MainScreen
+import com.simformsolutions.bagelandroid.ui.theme.BagelAndroidTheme
+import dagger.hilt.android.AndroidEntryPoint
+
+@AndroidEntryPoint
+class MainActivity : ComponentActivity() {
+
+ override fun onCreate(savedInstanceState: Bundle?) {
+ super.onCreate(savedInstanceState)
+ enableEdgeToEdge()
+ setContent {
+ BagelAndroidTheme {
+ Scaffold(
+ modifier = Modifier.fillMaxSize()
+ ) { innerPadding ->
+ MainApp(
+ modifier = Modifier
+ .padding(innerPadding),
+ )
+ }
+ }
+ }
+ }
+}
+
+@Composable
+private fun MainApp(
+ modifier: Modifier = Modifier,
+) {
+ MainRoute(
+ modifier = modifier
+ )
+}
diff --git a/android/app/src/main/java/com/simformsolutions/bagelandroid/ui/main/MainScreen.kt b/android/app/src/main/java/com/simformsolutions/bagelandroid/ui/main/MainScreen.kt
new file mode 100644
index 0000000..1ea9d3d
--- /dev/null
+++ b/android/app/src/main/java/com/simformsolutions/bagelandroid/ui/main/MainScreen.kt
@@ -0,0 +1,66 @@
+package com.simformsolutions.bagelandroid.ui.main
+
+import android.content.res.Configuration
+import androidx.compose.foundation.layout.Arrangement
+import androidx.compose.foundation.layout.Column
+import androidx.compose.foundation.layout.fillMaxSize
+import androidx.compose.foundation.layout.padding
+import androidx.compose.material3.Button
+import androidx.compose.material3.Scaffold
+import androidx.compose.material3.Text
+import androidx.compose.runtime.Composable
+import androidx.compose.ui.Alignment
+import androidx.compose.ui.Modifier
+import androidx.compose.ui.platform.LocalContext
+import androidx.compose.ui.tooling.preview.Preview
+import androidx.hilt.navigation.compose.hiltViewModel
+import com.simformsolutions.bagelandroid.ui.theme.BagelAndroidTheme
+
+@Composable
+fun MainRoute(modifier: Modifier = Modifier) {
+ val viewModel = hiltViewModel()
+
+ val context = LocalContext.current
+
+ MainScreen(
+ modifier = modifier,
+ onGetHotCoffee = viewModel::getHotCoffee,
+ )
+}
+
+@Composable
+fun MainScreen(
+ modifier: Modifier = Modifier,
+ onGetHotCoffee: () -> Unit,
+) {
+ Scaffold(
+ modifier = modifier
+ ) { innerPadding ->
+ Column(
+ modifier = Modifier
+ .padding(innerPadding)
+ .fillMaxSize(),
+ verticalArrangement = Arrangement.Center,
+ horizontalAlignment = Alignment.CenterHorizontally
+ ) {
+ Button(
+ onClick = onGetHotCoffee
+ ) {
+ Text("Get hot coffee")
+ }
+ }
+ }
+}
+
+@Preview(showBackground = true)
+@Preview(showBackground = true, uiMode = Configuration.UI_MODE_NIGHT_YES)
+@Composable
+private fun MainScreenPreview() {
+ BagelAndroidTheme {
+ MainScreen(
+ modifier = Modifier
+ .fillMaxSize(),
+ onGetHotCoffee = {},
+ )
+ }
+}
diff --git a/android/app/src/main/java/com/simformsolutions/bagelandroid/ui/main/MainViewModel.kt b/android/app/src/main/java/com/simformsolutions/bagelandroid/ui/main/MainViewModel.kt
new file mode 100644
index 0000000..2277db1
--- /dev/null
+++ b/android/app/src/main/java/com/simformsolutions/bagelandroid/ui/main/MainViewModel.kt
@@ -0,0 +1,32 @@
+package com.simformsolutions.bagelandroid.ui.main
+
+import androidx.lifecycle.ViewModel
+import androidx.lifecycle.viewModelScope
+import com.simformsolutions.bagelandroid.domain.repository.NetworkRepository
+import dagger.hilt.android.lifecycle.HiltViewModel
+import kotlinx.coroutines.Dispatchers
+import kotlinx.coroutines.flow.MutableSharedFlow
+import kotlinx.coroutines.flow.asSharedFlow
+import kotlinx.coroutines.launch
+import javax.inject.Inject
+
+@HiltViewModel
+class MainViewModel @Inject constructor(
+ private val networkRepository: NetworkRepository
+) : ViewModel() {
+
+ private val _resetCommand = MutableSharedFlow()
+ val resetCommand = _resetCommand.asSharedFlow()
+
+ fun getHotCoffee() {
+ viewModelScope.launch(Dispatchers.Default) {
+ networkRepository.getHotCoffee()
+ }
+ }
+
+ fun resetAndRestart() {
+ viewModelScope.launch {
+ _resetCommand.emit(Unit)
+ }
+ }
+}
\ No newline at end of file
diff --git a/android/app/src/main/java/com/simformsolutions/bagelandroid/ui/theme/Color.kt b/android/app/src/main/java/com/simformsolutions/bagelandroid/ui/theme/Color.kt
new file mode 100644
index 0000000..426d8bd
--- /dev/null
+++ b/android/app/src/main/java/com/simformsolutions/bagelandroid/ui/theme/Color.kt
@@ -0,0 +1,11 @@
+package com.simformsolutions.bagelandroid.ui.theme
+
+import androidx.compose.ui.graphics.Color
+
+val Purple80 = Color(0xFFD0BCFF)
+val PurpleGrey80 = Color(0xFFCCC2DC)
+val Pink80 = Color(0xFFEFB8C8)
+
+val Purple40 = Color(0xFF6650a4)
+val PurpleGrey40 = Color(0xFF625b71)
+val Pink40 = Color(0xFF7D5260)
\ No newline at end of file
diff --git a/android/app/src/main/java/com/simformsolutions/bagelandroid/ui/theme/Theme.kt b/android/app/src/main/java/com/simformsolutions/bagelandroid/ui/theme/Theme.kt
new file mode 100644
index 0000000..70e1391
--- /dev/null
+++ b/android/app/src/main/java/com/simformsolutions/bagelandroid/ui/theme/Theme.kt
@@ -0,0 +1,58 @@
+package com.simformsolutions.bagelandroid.ui.theme
+
+import android.app.Activity
+import android.os.Build
+import androidx.compose.foundation.isSystemInDarkTheme
+import androidx.compose.material3.MaterialTheme
+import androidx.compose.material3.darkColorScheme
+import androidx.compose.material3.dynamicDarkColorScheme
+import androidx.compose.material3.dynamicLightColorScheme
+import androidx.compose.material3.lightColorScheme
+import androidx.compose.runtime.Composable
+import androidx.compose.ui.platform.LocalContext
+
+private val DarkColorScheme = darkColorScheme(
+ primary = Purple80,
+ secondary = PurpleGrey80,
+ tertiary = Pink80
+)
+
+private val LightColorScheme = lightColorScheme(
+ primary = Purple40,
+ secondary = PurpleGrey40,
+ tertiary = Pink40
+
+ /* Other default colors to override
+ background = Color(0xFFFFFBFE),
+ surface = Color(0xFFFFFBFE),
+ onPrimary = Color.White,
+ onSecondary = Color.White,
+ onTertiary = Color.White,
+ onBackground = Color(0xFF1C1B1F),
+ onSurface = Color(0xFF1C1B1F),
+ */
+)
+
+@Composable
+fun BagelAndroidTheme(
+ darkTheme: Boolean = isSystemInDarkTheme(),
+ // Dynamic color is available on Android 12+
+ dynamicColor: Boolean = true,
+ content: @Composable () -> Unit
+) {
+ val colorScheme = when {
+ dynamicColor && Build.VERSION.SDK_INT >= Build.VERSION_CODES.S -> {
+ val context = LocalContext.current
+ if (darkTheme) dynamicDarkColorScheme(context) else dynamicLightColorScheme(context)
+ }
+
+ darkTheme -> DarkColorScheme
+ else -> LightColorScheme
+ }
+
+ MaterialTheme(
+ colorScheme = colorScheme,
+ typography = Typography,
+ content = content
+ )
+}
\ No newline at end of file
diff --git a/android/app/src/main/java/com/simformsolutions/bagelandroid/ui/theme/Type.kt b/android/app/src/main/java/com/simformsolutions/bagelandroid/ui/theme/Type.kt
new file mode 100644
index 0000000..a9a6bf6
--- /dev/null
+++ b/android/app/src/main/java/com/simformsolutions/bagelandroid/ui/theme/Type.kt
@@ -0,0 +1,34 @@
+package com.simformsolutions.bagelandroid.ui.theme
+
+import androidx.compose.material3.Typography
+import androidx.compose.ui.text.TextStyle
+import androidx.compose.ui.text.font.FontFamily
+import androidx.compose.ui.text.font.FontWeight
+import androidx.compose.ui.unit.sp
+
+// Set of Material typography styles to start with
+val Typography = Typography(
+ bodyLarge = TextStyle(
+ fontFamily = FontFamily.Default,
+ fontWeight = FontWeight.Normal,
+ fontSize = 16.sp,
+ lineHeight = 24.sp,
+ letterSpacing = 0.5.sp
+ )
+ /* Other default text styles to override
+ titleLarge = TextStyle(
+ fontFamily = FontFamily.Default,
+ fontWeight = FontWeight.Normal,
+ fontSize = 22.sp,
+ lineHeight = 28.sp,
+ letterSpacing = 0.sp
+ ),
+ labelSmall = TextStyle(
+ fontFamily = FontFamily.Default,
+ fontWeight = FontWeight.Medium,
+ fontSize = 11.sp,
+ lineHeight = 16.sp,
+ letterSpacing = 0.5.sp
+ )
+ */
+)
\ No newline at end of file
diff --git a/android/app/src/main/res/drawable/ic_launcher_background.xml b/android/app/src/main/res/drawable/ic_launcher_background.xml
new file mode 100644
index 0000000..07d5da9
--- /dev/null
+++ b/android/app/src/main/res/drawable/ic_launcher_background.xml
@@ -0,0 +1,170 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/android/app/src/main/res/drawable/ic_launcher_foreground.xml b/android/app/src/main/res/drawable/ic_launcher_foreground.xml
new file mode 100644
index 0000000..2b068d1
--- /dev/null
+++ b/android/app/src/main/res/drawable/ic_launcher_foreground.xml
@@ -0,0 +1,30 @@
+
+
+
+
+
+
+
+
+
+
+
\ No newline at end of file
diff --git a/android/app/src/main/res/mipmap-anydpi/ic_launcher.xml b/android/app/src/main/res/mipmap-anydpi/ic_launcher.xml
new file mode 100644
index 0000000..6f3b755
--- /dev/null
+++ b/android/app/src/main/res/mipmap-anydpi/ic_launcher.xml
@@ -0,0 +1,6 @@
+
+
+
+
+
+
\ No newline at end of file
diff --git a/android/app/src/main/res/mipmap-anydpi/ic_launcher_round.xml b/android/app/src/main/res/mipmap-anydpi/ic_launcher_round.xml
new file mode 100644
index 0000000..6f3b755
--- /dev/null
+++ b/android/app/src/main/res/mipmap-anydpi/ic_launcher_round.xml
@@ -0,0 +1,6 @@
+
+
+
+
+
+
\ No newline at end of file
diff --git a/android/app/src/main/res/mipmap-hdpi/ic_launcher.webp b/android/app/src/main/res/mipmap-hdpi/ic_launcher.webp
new file mode 100644
index 0000000..c209e78
Binary files /dev/null and b/android/app/src/main/res/mipmap-hdpi/ic_launcher.webp differ
diff --git a/android/app/src/main/res/mipmap-hdpi/ic_launcher_round.webp b/android/app/src/main/res/mipmap-hdpi/ic_launcher_round.webp
new file mode 100644
index 0000000..b2dfe3d
Binary files /dev/null and b/android/app/src/main/res/mipmap-hdpi/ic_launcher_round.webp differ
diff --git a/android/app/src/main/res/mipmap-mdpi/ic_launcher.webp b/android/app/src/main/res/mipmap-mdpi/ic_launcher.webp
new file mode 100644
index 0000000..4f0f1d6
Binary files /dev/null and b/android/app/src/main/res/mipmap-mdpi/ic_launcher.webp differ
diff --git a/android/app/src/main/res/mipmap-mdpi/ic_launcher_round.webp b/android/app/src/main/res/mipmap-mdpi/ic_launcher_round.webp
new file mode 100644
index 0000000..62b611d
Binary files /dev/null and b/android/app/src/main/res/mipmap-mdpi/ic_launcher_round.webp differ
diff --git a/android/app/src/main/res/mipmap-xhdpi/ic_launcher.webp b/android/app/src/main/res/mipmap-xhdpi/ic_launcher.webp
new file mode 100644
index 0000000..948a307
Binary files /dev/null and b/android/app/src/main/res/mipmap-xhdpi/ic_launcher.webp differ
diff --git a/android/app/src/main/res/mipmap-xhdpi/ic_launcher_round.webp b/android/app/src/main/res/mipmap-xhdpi/ic_launcher_round.webp
new file mode 100644
index 0000000..1b9a695
Binary files /dev/null and b/android/app/src/main/res/mipmap-xhdpi/ic_launcher_round.webp differ
diff --git a/android/app/src/main/res/mipmap-xxhdpi/ic_launcher.webp b/android/app/src/main/res/mipmap-xxhdpi/ic_launcher.webp
new file mode 100644
index 0000000..28d4b77
Binary files /dev/null and b/android/app/src/main/res/mipmap-xxhdpi/ic_launcher.webp differ
diff --git a/android/app/src/main/res/mipmap-xxhdpi/ic_launcher_round.webp b/android/app/src/main/res/mipmap-xxhdpi/ic_launcher_round.webp
new file mode 100644
index 0000000..9287f50
Binary files /dev/null and b/android/app/src/main/res/mipmap-xxhdpi/ic_launcher_round.webp differ
diff --git a/android/app/src/main/res/mipmap-xxxhdpi/ic_launcher.webp b/android/app/src/main/res/mipmap-xxxhdpi/ic_launcher.webp
new file mode 100644
index 0000000..aa7d642
Binary files /dev/null and b/android/app/src/main/res/mipmap-xxxhdpi/ic_launcher.webp differ
diff --git a/android/app/src/main/res/mipmap-xxxhdpi/ic_launcher_round.webp b/android/app/src/main/res/mipmap-xxxhdpi/ic_launcher_round.webp
new file mode 100644
index 0000000..9126ae3
Binary files /dev/null and b/android/app/src/main/res/mipmap-xxxhdpi/ic_launcher_round.webp differ
diff --git a/android/app/src/main/res/values/colors.xml b/android/app/src/main/res/values/colors.xml
new file mode 100644
index 0000000..f8c6127
--- /dev/null
+++ b/android/app/src/main/res/values/colors.xml
@@ -0,0 +1,10 @@
+
+
+ #FFBB86FC
+ #FF6200EE
+ #FF3700B3
+ #FF03DAC5
+ #FF018786
+ #FF000000
+ #FFFFFFFF
+
\ No newline at end of file
diff --git a/android/app/src/main/res/values/strings.xml b/android/app/src/main/res/values/strings.xml
new file mode 100644
index 0000000..7ce6977
--- /dev/null
+++ b/android/app/src/main/res/values/strings.xml
@@ -0,0 +1,3 @@
+
+ BagelAndroid
+
\ No newline at end of file
diff --git a/android/app/src/main/res/values/themes.xml b/android/app/src/main/res/values/themes.xml
new file mode 100644
index 0000000..448f151
--- /dev/null
+++ b/android/app/src/main/res/values/themes.xml
@@ -0,0 +1,5 @@
+
+
+
+
+
\ No newline at end of file
diff --git a/android/app/src/main/res/xml/backup_rules.xml b/android/app/src/main/res/xml/backup_rules.xml
new file mode 100644
index 0000000..fa0f996
--- /dev/null
+++ b/android/app/src/main/res/xml/backup_rules.xml
@@ -0,0 +1,13 @@
+
+
+
+
\ No newline at end of file
diff --git a/android/app/src/main/res/xml/data_extraction_rules.xml b/android/app/src/main/res/xml/data_extraction_rules.xml
new file mode 100644
index 0000000..9ee9997
--- /dev/null
+++ b/android/app/src/main/res/xml/data_extraction_rules.xml
@@ -0,0 +1,19 @@
+
+
+
+
+
+
+
\ No newline at end of file
diff --git a/android/app/src/test/java/com/simformsolutions/bagelandroid/ExampleUnitTest.kt b/android/app/src/test/java/com/simformsolutions/bagelandroid/ExampleUnitTest.kt
new file mode 100644
index 0000000..342f551
--- /dev/null
+++ b/android/app/src/test/java/com/simformsolutions/bagelandroid/ExampleUnitTest.kt
@@ -0,0 +1,17 @@
+package com.simformsolutions.bagelandroid
+
+import org.junit.Test
+
+import org.junit.Assert.*
+
+/**
+ * Example local unit test, which will execute on the development machine (host).
+ *
+ * See [testing documentation](http://d.android.com/tools/testing).
+ */
+class ExampleUnitTest {
+ @Test
+ fun addition_isCorrect() {
+ assertEquals(4, 2 + 2)
+ }
+}
\ No newline at end of file
diff --git a/android/bagel/.gitignore b/android/bagel/.gitignore
new file mode 100644
index 0000000..7001498
--- /dev/null
+++ b/android/bagel/.gitignore
@@ -0,0 +1,57 @@
+# Built application files
+*.apk
+*.ap_
+
+# Files for the ART/Dalvik VM
+*.dex
+
+# Java class files
+*.class
+
+# Generated files
+bin/
+gen/
+out/
+
+# Gradle files
+/.idea
+.gradle/
+build/
+
+# Local configuration file (sdk path, etc)
+local.properties
+
+# Proguard folder generated by Eclipse
+proguard/
+
+# Log Files
+*.log
+
+# Android Studio Navigation editor temp files
+.navigation/
+
+# Android Studio captures folder
+captures/
+
+# Intellij
+*.iml
+.idea/workspace.xml
+.idea/tasks.xml
+.idea/gradle.xml
+.idea/dictionaries
+.idea/libraries
+app/.idea/
+
+# Mac
+*.DS_Store
+
+# Keystore files
+*.jks
+
+# External native build folder generated in Android Studio 2.2 and later
+.externalNativeBuild
+
+# Temporary API docs
+docs/api
+
+*.gpg
diff --git a/android/bagel/build.gradle.kts b/android/bagel/build.gradle.kts
new file mode 100644
index 0000000..43e7fd9
--- /dev/null
+++ b/android/bagel/build.gradle.kts
@@ -0,0 +1,96 @@
+import com.vanniktech.maven.publish.AndroidSingleVariantLibrary
+import com.vanniktech.maven.publish.SonatypeHost
+
+plugins {
+ alias(libs.plugins.android.library)
+ alias(libs.plugins.jetbrains.kotlin.android)
+ alias(libs.plugins.kotlinx.serialization)
+ alias(libs.plugins.vanniktechMavenPublish)
+}
+
+mavenPublishing {
+ coordinates("com.simform", "bagel", "1.0.1")
+ publishToMavenCentral(SonatypeHost.CENTRAL_PORTAL, automaticRelease = false)
+ configure(AndroidSingleVariantLibrary())
+
+ pom {
+ packaging = "aar"
+ name.set("Bagel")
+ description.set("Bagel is a little native iOS/Android network debugger")
+ url.set("https://github.com/mobile-simformsolutions/Bagel.git")
+ inceptionYear.set("2024")
+
+ licenses {
+ license {
+ name.set("Apache License Version 2.0, January 2004")
+ url.set("https://github.com/mobile-simformsolutions/Bagel?tab=License-1-ov-file")
+ }
+ }
+
+ developers {
+ developer {
+ id.set("simform")
+ name.set("Simform")
+ email.set("developer@simform.com")
+ }
+ }
+
+ scm {
+ connection.set("scm:git@github.com:mobile-simformsolutions/Bagel")
+ developerConnection.set("scm:git@github.com:mobile-simformsolutions/Bagel.git")
+ url.set("https://github.com/mobile-simformsolutions/Bagel.git")
+ }
+ }
+
+ signAllPublications()
+}
+
+android {
+ namespace = "com.simform.bagel"
+ compileSdk = libs.versions.compileSdk.get().toInt()
+
+ defaultConfig {
+ minSdk = libs.versions.minSdk.get().toInt()
+ compileSdk = libs.versions.compileSdk.get().toInt()
+
+ aarMetadata {
+ minCompileSdk = libs.versions.minSdk.get().toInt()
+ }
+
+ multiDexEnabled = true
+
+ testInstrumentationRunner = "androidx.test.runner.AndroidJUnitRunner"
+ consumerProguardFiles("consumer-rules.pro")
+ }
+
+ buildTypes {
+ release {
+ isMinifyEnabled = true
+ proguardFiles(
+ getDefaultProguardFile("proguard-android-optimize.txt"),
+ "proguard-rules.pro"
+ )
+ }
+ }
+ compileOptions {
+ sourceCompatibility = JavaVersion.VERSION_1_8
+ targetCompatibility = JavaVersion.VERSION_1_8
+ }
+ kotlinOptions {
+ jvmTarget = "1.8"
+ }
+}
+
+dependencies {
+ implementation(libs.androidx.core.ktx)
+ implementation(libs.androidx.appcompat)
+ implementation(libs.material)
+ implementation(libs.androidx.startup.runtime)
+ implementation(libs.kotlinx.serialization.json)
+ implementation(libs.logging.interceptor)
+ implementation(libs.timber)
+ implementation(libs.socket.io.client)
+ testImplementation(libs.junit)
+ androidTestImplementation(libs.androidx.junit)
+ androidTestImplementation(libs.androidx.espresso.core)
+}
\ No newline at end of file
diff --git a/android/bagel/consumer-rules.pro b/android/bagel/consumer-rules.pro
new file mode 100644
index 0000000..e69de29
diff --git a/android/bagel/proguard-rules.pro b/android/bagel/proguard-rules.pro
new file mode 100644
index 0000000..e630acc
--- /dev/null
+++ b/android/bagel/proguard-rules.pro
@@ -0,0 +1,15 @@
+-keep class com.simform.bagel.Bagel {
+ public *;
+}
+-keep class com.simform.bagel.intercept.BagelInterceptor {
+ public *;
+}
+-keep class com.simform.bagel.intercept.BagelInterceptor$Companion {
+ public *;
+}
+-keep class com.simform.bagel.config.BagelConfiguration {
+ public *;
+}
+-keep class com.simform.bagel.config.BagelConfiguration$Companion {
+ public *;
+}
\ No newline at end of file
diff --git a/android/bagel/src/main/AndroidManifest.xml b/android/bagel/src/main/AndroidManifest.xml
new file mode 100644
index 0000000..a5918e6
--- /dev/null
+++ b/android/bagel/src/main/AndroidManifest.xml
@@ -0,0 +1,4 @@
+
+
+
+
\ No newline at end of file
diff --git a/android/bagel/src/main/java/com/simform/bagel/Bagel.kt b/android/bagel/src/main/java/com/simform/bagel/Bagel.kt
new file mode 100644
index 0000000..dabe908
--- /dev/null
+++ b/android/bagel/src/main/java/com/simform/bagel/Bagel.kt
@@ -0,0 +1,31 @@
+package com.simform.bagel
+
+import android.content.Context
+import com.simform.bagel.browser.BagelBrowser
+import com.simform.bagel.config.BagelConfiguration
+import com.simform.bagel.model.Device
+import com.simform.bagel.model.Project
+import kotlinx.coroutines.Dispatchers
+import kotlinx.coroutines.MainScope
+import kotlinx.coroutines.launch
+
+object Bagel {
+ internal const val TAG = "Bagel"
+
+ private val mainScope = MainScope()
+
+ @JvmStatic
+ @JvmOverloads
+ fun start(
+ context: Context,
+ bagelConfiguration: BagelConfiguration = BagelConfiguration.getDefault(context)
+ ) {
+ mainScope.launch(Dispatchers.Default) {
+ // Initialize models
+ Device.initialize(context = context)
+ Project.initialize(context = context, bagelConfiguration = bagelConfiguration)
+ // Initialize network browser
+ BagelBrowser.initialize(context = context, bagelConfiguration = bagelConfiguration)
+ }
+ }
+}
\ No newline at end of file
diff --git a/android/bagel/src/main/java/com/simform/bagel/browser/BagelBrowser.kt b/android/bagel/src/main/java/com/simform/bagel/browser/BagelBrowser.kt
new file mode 100644
index 0000000..6061b96
--- /dev/null
+++ b/android/bagel/src/main/java/com/simform/bagel/browser/BagelBrowser.kt
@@ -0,0 +1,27 @@
+package com.simform.bagel.browser
+
+import android.content.Context
+import com.simform.bagel.config.BagelConfiguration
+import com.simform.bagel.model.RequestInfo
+
+internal interface BagelBrowser {
+
+ fun sendPacket(requestInfo: RequestInfo)
+
+ companion object {
+ @Volatile
+ private var INSTANCE: BagelBrowser? = null
+
+ @Synchronized
+ fun initialize(context: Context, bagelConfiguration: BagelConfiguration) {
+ INSTANCE = INSTANCE ?: BagelBrowserImpl.getInstance(
+ context = context,
+ bagelConfiguration = bagelConfiguration
+ )
+ }
+
+ @Synchronized
+ fun getInstance(): BagelBrowser =
+ INSTANCE!!
+ }
+}
diff --git a/android/bagel/src/main/java/com/simform/bagel/browser/BagelBrowserImpl.kt b/android/bagel/src/main/java/com/simform/bagel/browser/BagelBrowserImpl.kt
new file mode 100644
index 0000000..40e18c8
--- /dev/null
+++ b/android/bagel/src/main/java/com/simform/bagel/browser/BagelBrowserImpl.kt
@@ -0,0 +1,245 @@
+package com.simform.bagel.browser
+
+import android.content.Context
+import android.net.nsd.NsdManager
+import android.net.nsd.NsdServiceInfo
+import android.os.Build
+import androidx.annotation.RequiresApi
+import com.simform.bagel.config.BagelConfiguration
+import com.simform.bagel.model.Packet
+import com.simform.bagel.model.RequestInfo
+import com.simform.bagel.nsd.NsdDiscoveryListener
+import com.simform.bagel.nsd.NsdResolveListener
+import com.simform.bagel.nsd.NsdServiceInfoCallback
+import kotlinx.coroutines.Dispatchers
+import kotlinx.coroutines.MainScope
+import kotlinx.coroutines.async
+import kotlinx.coroutines.awaitAll
+import kotlinx.coroutines.launch
+import kotlinx.serialization.encodeToString
+import kotlinx.serialization.json.Json
+import timber.log.Timber
+import java.net.Socket
+import java.nio.ByteBuffer
+import java.nio.ByteOrder
+import java.util.concurrent.ConcurrentHashMap
+import java.util.concurrent.Executors
+
+internal class BagelBrowserImpl private constructor(
+ private val context: Context,
+ private val bagelConfiguration: BagelConfiguration
+) : BagelBrowser {
+
+ private val bagelScope = MainScope()
+
+ private val json by lazy {
+ Json {
+ prettyPrint = false
+ ignoreUnknownKeys = true
+ encodeDefaults = true
+ }
+ }
+
+ private val nsdManager by lazy {
+ context.getSystemService(Context.NSD_SERVICE) as NsdManager
+ }
+
+ private val discoverExecutor by lazy { Executors.newSingleThreadExecutor() }
+
+ private val nsdServices = ConcurrentHashMap()
+ private val socketConnections = ConcurrentHashMap>()
+
+ private val nsdServiceInfoCallback
+ get() = @RequiresApi(Build.VERSION_CODES.UPSIDE_DOWN_CAKE)
+ object : NsdServiceInfoCallback {
+
+ private var nsdServiceInfo: NsdServiceInfo? = null
+
+ override fun onServiceUpdated(serviceInfo: NsdServiceInfo) {
+ super.onServiceUpdated(serviceInfo)
+ nsdServiceInfo = serviceInfo
+ onNsdServiceInfoFound(serviceInfo)
+ }
+
+ override fun onServiceLost() {
+ super.onServiceLost()
+ val serviceInfo = nsdServiceInfo ?: return
+ onNsdServiceLost(serviceInfo)
+ }
+ }
+
+ private val nsdResolveListener
+ get() = object : NsdResolveListener {
+
+ private var nsdServiceInfo: NsdServiceInfo? = null
+
+ override fun onServiceResolved(serviceInfo: NsdServiceInfo?) {
+ super.onServiceResolved(serviceInfo)
+ val nsdInfo = nsdServiceInfo
+ if (serviceInfo == null && nsdInfo != null) {
+ nsdServices.remove(nsdInfo.serviceName)
+ return
+ }
+ serviceInfo ?: return
+ nsdServiceInfo = serviceInfo
+ onNsdServiceInfoFound(serviceInfo)
+ }
+ }
+
+ private val nsdDiscoveryListener = object : NsdDiscoveryListener {
+ override fun onServiceFound(serviceInfo: NsdServiceInfo?) {
+ super.onServiceFound(serviceInfo)
+
+ serviceInfo ?: return
+
+ if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.UPSIDE_DOWN_CAKE) {
+ nsdManager.registerServiceInfoCallback(
+ serviceInfo,
+ Executors.newSingleThreadExecutor(),
+ nsdServiceInfoCallback
+ )
+ } else {
+ nsdManager.resolveService(
+ serviceInfo,
+ nsdResolveListener
+ )
+ }
+ }
+
+ override fun onServiceLost(serviceInfo: NsdServiceInfo?) {
+ super.onServiceLost(serviceInfo)
+ serviceInfo ?: return
+ onNsdServiceLost(serviceInfo)
+ }
+ }
+
+ override fun sendPacket(requestInfo: RequestInfo) {
+ val packet = Packet(requestInfo = requestInfo)
+ .let { json.encodeToString(it) }
+ .toByteArray()
+ val buffer = ByteBuffer.allocate(8 + packet.size)
+ .order(ByteOrder.LITTLE_ENDIAN)
+ .apply {
+ putLong(packet.size.toLong())
+ put(packet)
+ }
+ bagelScope.launch(Dispatchers.IO) {
+ socketConnections.values.flatMap { connection ->
+ connection.map { (address, socket) ->
+ async {
+ try {
+ if (!socket.isClosed && socket.isConnected) {
+ socket.getOutputStream()
+ .write(buffer.array())
+ }
+ } catch (e: Exception) {
+ Timber.w(message = "Failed to write data for $address", t = e)
+ }
+ }
+ }
+ }.awaitAll()
+ }
+ }
+
+ init {
+ start()
+ }
+
+ private fun start() = with(nsdManager) {
+ if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.TIRAMISU) {
+ discoverServices(
+ bagelConfiguration.netServiceType,
+ NsdManager.PROTOCOL_DNS_SD,
+ null,
+ discoverExecutor,
+ nsdDiscoveryListener
+ )
+ } else {
+ discoverServices(
+ bagelConfiguration.netServiceType,
+ NsdManager.PROTOCOL_DNS_SD,
+ nsdDiscoveryListener
+ )
+ }
+ }
+
+ private fun onNsdServiceInfoFound(nsdServiceInfo: NsdServiceInfo) {
+ bagelScope.launch(Dispatchers.IO) {
+ val serviceName = nsdServiceInfo.serviceName
+ nsdServices[serviceName] = nsdServiceInfo
+
+ val addresses = if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.UPSIDE_DOWN_CAKE) {
+ nsdServiceInfo.hostAddresses
+ } else {
+ listOf(nsdServiceInfo.host)
+ }
+
+ val socketConnectionsOfThisService =
+ socketConnections.getOrDefault(serviceName, mutableMapOf())
+ val updatedConnections = mutableMapOf()
+
+ addresses.forEach { address ->
+ val hostAddress = address.hostAddress
+ if (hostAddress != null) {
+ val hostConnectionExists =
+ socketConnectionsOfThisService.containsKey(hostAddress)
+ if (!hostConnectionExists) {
+ try {
+ Timber.d("Connecting to $hostAddress:${nsdServiceInfo.port}")
+ val socket = Socket(hostAddress, nsdServiceInfo.port).apply {
+ keepAlive = true
+ }
+
+ updatedConnections[hostAddress] = socket
+
+ if (socket.isConnected) {
+ Timber.d("Connected with $hostAddress:${nsdServiceInfo.port} hostname: $serviceName")
+ }
+ } catch (e: Exception) {
+ Timber.w(e)
+ }
+ } else {
+ // We're sure that it exists so lets copy it over
+ updatedConnections[hostAddress] =
+ socketConnectionsOfThisService[hostAddress]!!
+ }
+ }
+ }
+
+ socketConnections[serviceName] = updatedConnections
+
+ // Close socket connection with addresses that no longer exist in bagel service
+ (socketConnections.keys - updatedConnections.keys)
+ .mapNotNull { key -> socketConnectionsOfThisService[key] }
+ .forEach { socket ->
+ if (socket.isConnected) {
+ socket.close()
+ }
+ }
+ }
+ }
+
+ private fun onNsdServiceLost(nsdServiceInfo: NsdServiceInfo) {
+ val serviceName = nsdServiceInfo.serviceName
+ if (!nsdServices.containsKey(serviceName)) {
+ return
+ }
+ socketConnections[serviceName]?.values?.forEach { socket ->
+ if (socket.isConnected) {
+ socket.close()
+ }
+ }
+ socketConnections.remove(serviceName)
+ nsdServices.remove(nsdServiceInfo.serviceName)
+ }
+
+ companion object {
+ fun getInstance(
+ context: Context,
+ bagelConfiguration: BagelConfiguration
+ ): BagelBrowserImpl = BagelBrowserImpl(
+ context = context,
+ bagelConfiguration = bagelConfiguration
+ )
+ }
+}
diff --git a/android/bagel/src/main/java/com/simform/bagel/config/BagelConfiguration.kt b/android/bagel/src/main/java/com/simform/bagel/config/BagelConfiguration.kt
new file mode 100644
index 0000000..e467a7b
--- /dev/null
+++ b/android/bagel/src/main/java/com/simform/bagel/config/BagelConfiguration.kt
@@ -0,0 +1,17 @@
+package com.simform.bagel.config
+
+import android.content.Context
+
+data class BagelConfiguration(
+ val projectName: String,
+ val netServiceType: String = SERVICE_TYPE,
+) {
+ companion object {
+ private const val SERVICE_TYPE = "_Bagel._tcp"
+
+ @JvmStatic
+ fun getDefault(context: Context): BagelConfiguration = BagelConfiguration(
+ projectName = context.packageManager.getApplicationLabel(context.applicationInfo).toString()
+ )
+ }
+}
diff --git a/android/bagel/src/main/java/com/simform/bagel/intercept/BagelInterceptor.kt b/android/bagel/src/main/java/com/simform/bagel/intercept/BagelInterceptor.kt
new file mode 100644
index 0000000..f91f54c
--- /dev/null
+++ b/android/bagel/src/main/java/com/simform/bagel/intercept/BagelInterceptor.kt
@@ -0,0 +1,64 @@
+package com.simform.bagel.intercept
+
+import com.simform.bagel.browser.BagelBrowser
+import com.simform.bagel.model.RequestInfo
+import okhttp3.Interceptor
+import okhttp3.RequestBody
+import okhttp3.Response
+import okio.Buffer
+import timber.log.Timber
+import java.util.Base64
+
+class BagelInterceptor private constructor() : Interceptor {
+
+ private val bagelBrowser by lazy {
+ BagelBrowser.getInstance()
+ }
+
+ override fun intercept(chain: Interceptor.Chain): Response =
+ chain.proceed(chain.request()).also {
+ bagelBrowser.sendPacket(requestInfo = it.requestInfo())
+ }
+
+ private fun Response.requestInfo(): RequestInfo = RequestInfo(
+ url = request.url.toString(),
+ requestMethod = request.method,
+ requestHeaders = request.headers.toMap(),
+ startDate = sentRequestAtMillis / 1000,
+ endDate = receivedResponseAtMillis / 1000,
+ responseData = responseBase64(),
+ requestBody = requestBase64(),
+ statusCode = code.toString()
+ )
+
+ private fun Response.responseBase64(): String = Base64.getEncoder().encodeToString(
+ peekBody(Long.MAX_VALUE).bytes()
+ )
+
+ private fun Response.requestBase64(): String = request.body?.toByteArray()?.let {
+ Base64.getEncoder().encodeToString(it)
+ }.orEmpty()
+
+ private fun RequestBody.toByteArray(): ByteArray? = try {
+ val buffer = Buffer().apply {
+ writeTo(this)
+ }
+ buffer.readByteArray()
+ } catch (e: Exception) {
+ Timber.e(e)
+ null
+ }
+
+ companion object {
+ @Volatile
+ private var INSTANCE: BagelInterceptor? = null
+
+ @JvmStatic
+ @Synchronized
+ fun getInstance(): BagelInterceptor =
+ INSTANCE ?: let {
+ INSTANCE = BagelInterceptor()
+ INSTANCE!!
+ }
+ }
+}
\ No newline at end of file
diff --git a/android/bagel/src/main/java/com/simform/bagel/model/Device.kt b/android/bagel/src/main/java/com/simform/bagel/model/Device.kt
new file mode 100644
index 0000000..3b76ca7
--- /dev/null
+++ b/android/bagel/src/main/java/com/simform/bagel/model/Device.kt
@@ -0,0 +1,33 @@
+package com.simform.bagel.model
+
+import android.content.Context
+import android.os.Build
+import kotlinx.serialization.SerialName
+import kotlinx.serialization.Serializable
+
+@Serializable
+internal data class Device(
+ @SerialName("deviceDescription")
+ val deviceDescription: String,
+ @SerialName("deviceName")
+ val deviceName: String,
+ @SerialName("deviceId")
+ val deviceId: String,
+) {
+ internal companion object {
+ @Volatile
+ private var INSTANCE: Device? = null
+
+ @Synchronized
+ fun initialize(context: Context) {
+ INSTANCE = Device(
+ deviceName = Build.MODEL,
+ deviceDescription = "Android " + Build.VERSION.RELEASE,
+ deviceId = "${Build.MANUFACTURER}-${Build.MODEL}-android-${Build.VERSION.RELEASE}"
+ )
+ }
+
+ @Synchronized
+ fun getInstance(): Device = INSTANCE!!
+ }
+}
diff --git a/android/bagel/src/main/java/com/simform/bagel/model/Packet.kt b/android/bagel/src/main/java/com/simform/bagel/model/Packet.kt
new file mode 100644
index 0000000..cd9edb4
--- /dev/null
+++ b/android/bagel/src/main/java/com/simform/bagel/model/Packet.kt
@@ -0,0 +1,17 @@
+package com.simform.bagel.model
+
+import kotlinx.serialization.SerialName
+import kotlinx.serialization.Serializable
+import java.util.UUID
+
+@Serializable
+internal data class Packet(
+ @SerialName("device")
+ val device: Device = Device.getInstance(),
+ @SerialName("packetId")
+ val packetId: String = UUID.randomUUID().toString(),
+ @SerialName("requestInfo")
+ val requestInfo: RequestInfo,
+ @SerialName("project")
+ val project: Project = Project.getInstance()
+)
diff --git a/android/bagel/src/main/java/com/simform/bagel/model/Project.kt b/android/bagel/src/main/java/com/simform/bagel/model/Project.kt
new file mode 100644
index 0000000..bad444e
--- /dev/null
+++ b/android/bagel/src/main/java/com/simform/bagel/model/Project.kt
@@ -0,0 +1,25 @@
+package com.simform.bagel.model
+
+import android.content.Context
+import com.simform.bagel.config.BagelConfiguration
+import kotlinx.serialization.SerialName
+import kotlinx.serialization.Serializable
+
+@Serializable
+internal data class Project(
+ @SerialName("projectName")
+ val projectName: String
+) {
+ internal companion object {
+ @Volatile
+ private var INSTANCE: Project? = null
+
+ @Synchronized
+ fun initialize(context: Context, bagelConfiguration: BagelConfiguration) {
+ INSTANCE = Project(projectName = bagelConfiguration.projectName)
+ }
+
+ @Synchronized
+ fun getInstance(): Project = INSTANCE!!
+ }
+}
diff --git a/android/bagel/src/main/java/com/simform/bagel/model/RequestInfo.kt b/android/bagel/src/main/java/com/simform/bagel/model/RequestInfo.kt
new file mode 100644
index 0000000..55a9b6a
--- /dev/null
+++ b/android/bagel/src/main/java/com/simform/bagel/model/RequestInfo.kt
@@ -0,0 +1,24 @@
+package com.simform.bagel.model
+
+import kotlinx.serialization.SerialName
+import kotlinx.serialization.Serializable
+
+@Serializable
+internal data class RequestInfo(
+ @SerialName("url")
+ val url: String,
+ @SerialName("responseData")
+ val responseData: String,
+ @SerialName("requestBody")
+ val requestBody: String,
+ @SerialName("endDate")
+ val endDate: Long,
+ @SerialName("requestHeaders")
+ val requestHeaders: Map,
+ @SerialName("requestMethod")
+ val requestMethod: String,
+ @SerialName("statusCode")
+ val statusCode: String,
+ @SerialName("startDate")
+ val startDate: Long
+)
diff --git a/android/bagel/src/main/java/com/simform/bagel/nsd/NsdDiscoveryListener.kt b/android/bagel/src/main/java/com/simform/bagel/nsd/NsdDiscoveryListener.kt
new file mode 100644
index 0000000..0d88388
--- /dev/null
+++ b/android/bagel/src/main/java/com/simform/bagel/nsd/NsdDiscoveryListener.kt
@@ -0,0 +1,32 @@
+package com.simform.bagel.nsd
+
+import android.net.nsd.NsdManager
+import android.net.nsd.NsdServiceInfo
+import com.simform.bagel.Bagel.TAG
+import timber.log.Timber
+
+internal interface NsdDiscoveryListener : NsdManager.DiscoveryListener {
+ override fun onStartDiscoveryFailed(serviceType: String?, errorCode: Int) {
+ Timber.tag(TAG).d("onStartDiscoveryFailed for $serviceType with errorCode $errorCode")
+ }
+
+ override fun onStopDiscoveryFailed(serviceType: String?, errorCode: Int) {
+ Timber.tag(TAG).d("onStopDiscoveryFailed for $serviceType with errorCode $errorCode")
+ }
+
+ override fun onDiscoveryStarted(serviceType: String?) {
+ Timber.tag(TAG).d("onDiscoveryStarted for $serviceType")
+ }
+
+ override fun onDiscoveryStopped(serviceType: String?) {
+ Timber.tag(TAG).d("onDiscoveryStopped for $serviceType")
+ }
+
+ override fun onServiceFound(serviceInfo: NsdServiceInfo?) {
+ Timber.tag(TAG).d("onServiceFound for $serviceInfo")
+ }
+
+ override fun onServiceLost(serviceInfo: NsdServiceInfo?) {
+ Timber.tag(TAG).d("onServiceLost for $serviceInfo")
+ }
+}
\ No newline at end of file
diff --git a/android/bagel/src/main/java/com/simform/bagel/nsd/NsdResolveListener.kt b/android/bagel/src/main/java/com/simform/bagel/nsd/NsdResolveListener.kt
new file mode 100644
index 0000000..3d90531
--- /dev/null
+++ b/android/bagel/src/main/java/com/simform/bagel/nsd/NsdResolveListener.kt
@@ -0,0 +1,16 @@
+package com.simform.bagel.nsd
+
+import android.net.nsd.NsdManager
+import android.net.nsd.NsdServiceInfo
+import com.simform.bagel.Bagel.TAG
+import timber.log.Timber
+
+internal interface NsdResolveListener : NsdManager.ResolveListener {
+ override fun onResolveFailed(serviceInfo: NsdServiceInfo?, errorCode: Int) {
+ Timber.tag(TAG).d("onResolveFailed for $serviceInfo with errorCode $errorCode")
+ }
+
+ override fun onServiceResolved(serviceInfo: NsdServiceInfo?) {
+ Timber.tag(TAG).d("onServiceResolved for $serviceInfo")
+ }
+}
\ No newline at end of file
diff --git a/android/bagel/src/main/java/com/simform/bagel/nsd/NsdServiceInfoCallback.kt b/android/bagel/src/main/java/com/simform/bagel/nsd/NsdServiceInfoCallback.kt
new file mode 100644
index 0000000..3ae398d
--- /dev/null
+++ b/android/bagel/src/main/java/com/simform/bagel/nsd/NsdServiceInfoCallback.kt
@@ -0,0 +1,27 @@
+package com.simform.bagel.nsd
+
+import android.net.nsd.NsdManager
+import android.net.nsd.NsdServiceInfo
+import android.os.Build
+import androidx.annotation.RequiresApi
+import com.simform.bagel.Bagel.TAG
+import timber.log.Timber
+
+@RequiresApi(Build.VERSION_CODES.UPSIDE_DOWN_CAKE)
+internal interface NsdServiceInfoCallback : NsdManager.ServiceInfoCallback {
+ override fun onServiceInfoCallbackRegistrationFailed(errorCode: Int) {
+ Timber.tag(TAG).d("onServiceInfoCallbackRegistrationFailed with errorCode $errorCode")
+ }
+
+ override fun onServiceUpdated(serviceInfo: NsdServiceInfo) {
+ Timber.tag(TAG).d("onServiceUpdated for $serviceInfo")
+ }
+
+ override fun onServiceLost() {
+ Timber.tag(TAG).d("onServiceLost")
+ }
+
+ override fun onServiceInfoCallbackUnregistered() {
+ Timber.tag(TAG).d("onServiceInfoCallbackUnregistered")
+ }
+}
\ No newline at end of file
diff --git a/android/build.gradle.kts b/android/build.gradle.kts
new file mode 100644
index 0000000..23e2fac
--- /dev/null
+++ b/android/build.gradle.kts
@@ -0,0 +1,9 @@
+// Top-level build file where you can add configuration options common to all sub-projects/modules.
+plugins {
+ alias(libs.plugins.android.application) apply false
+ alias(libs.plugins.jetbrains.kotlin.android) apply false
+ alias(libs.plugins.compose.compiler) apply false
+ alias(libs.plugins.hilt) apply false
+ alias(libs.plugins.ksp) apply false
+ alias(libs.plugins.android.library) apply false
+}
\ No newline at end of file
diff --git a/android/gradle.defaults.properties b/android/gradle.defaults.properties
new file mode 100644
index 0000000..4389c66
--- /dev/null
+++ b/android/gradle.defaults.properties
@@ -0,0 +1,30 @@
+# Project-wide Gradle settings.
+# IDE (e.g. Android Studio) users:
+# Gradle settings configured through the IDE *will override*
+# any settings specified in this file.
+# For more details on how to configure your build environment visit
+# http://www.gradle.org/docs/current/userguide/build_environment.html
+# Specifies the JVM arguments used for the daemon process.
+# The setting is particularly useful for tweaking memory settings.
+org.gradle.jvmargs=-Xmx2048m -Dfile.encoding=UTF-8
+# When configured, Gradle will run in incubating parallel mode.
+# This option should only be used with decoupled projects. For more details, visit
+# https://developer.android.com/r/tools/gradle-multi-project-decoupled-projects
+# org.gradle.parallel=true
+# AndroidX package structure to make it clearer which packages are bundled with the
+# Android operating system, and which are packaged with your app's APK
+# https://developer.android.com/topic/libraries/support-library/androidx-rn
+android.useAndroidX=true
+# Kotlin code style for this project: "official" or "obsolete":
+kotlin.code.style=official
+# Enables namespacing of each library's R class so that its R class includes only the
+# resources declared in the library itself and none from the library's dependencies,
+# thereby reducing the size of the R class for that library
+android.nonTransitiveRClass=true
+
+mavenCentralUsername=
+mavenCentralPassword=
+
+signing.keyId=0x00000000
+signing.password=
+signing.secretKeyRingFile=
\ No newline at end of file
diff --git a/android/gradle.properties b/android/gradle.properties
new file mode 100644
index 0000000..20e2a01
--- /dev/null
+++ b/android/gradle.properties
@@ -0,0 +1,23 @@
+# Project-wide Gradle settings.
+# IDE (e.g. Android Studio) users:
+# Gradle settings configured through the IDE *will override*
+# any settings specified in this file.
+# For more details on how to configure your build environment visit
+# http://www.gradle.org/docs/current/userguide/build_environment.html
+# Specifies the JVM arguments used for the daemon process.
+# The setting is particularly useful for tweaking memory settings.
+org.gradle.jvmargs=-Xmx2048m -Dfile.encoding=UTF-8
+# When configured, Gradle will run in incubating parallel mode.
+# This option should only be used with decoupled projects. For more details, visit
+# https://developer.android.com/r/tools/gradle-multi-project-decoupled-projects
+# org.gradle.parallel=true
+# AndroidX package structure to make it clearer which packages are bundled with the
+# Android operating system, and which are packaged with your app's APK
+# https://developer.android.com/topic/libraries/support-library/androidx-rn
+android.useAndroidX=true
+# Kotlin code style for this project: "official" or "obsolete":
+kotlin.code.style=official
+# Enables namespacing of each library's R class so that its R class includes only the
+# resources declared in the library itself and none from the library's dependencies,
+# thereby reducing the size of the R class for that library
+android.nonTransitiveRClass=true
\ No newline at end of file
diff --git a/android/gradle/libs.versions.toml b/android/gradle/libs.versions.toml
new file mode 100644
index 0000000..233bc5c
--- /dev/null
+++ b/android/gradle/libs.versions.toml
@@ -0,0 +1,69 @@
+[versions]
+minSdk = "26"
+targetSdk ="34"
+compileSdk = "34"
+
+agp = "8.4.0"
+kotlin = "2.0.0"
+coreKtx = "1.13.1"
+junit = "4.13.2"
+junitVersion = "1.1.5"
+espressoCore = "3.5.1"
+lifecycleRuntimeKtx = "2.8.0"
+activityCompose = "1.9.0"
+composeBom = "2024.05.00"
+timber = "5.0.1"
+lifecycleRuntimeCompose = "2.8.0"
+kotlinx-serialization-json = "1.6.3"
+kotlinx-serialization-plugin = "2.0.0"
+startupRuntime = "1.1.1"
+retrofitBom = "2.11.0"
+hilt = "2.51.1"
+ksp = "2.0.0-1.0.21"
+loggingInterceptor = "5.0.0-alpha.10"
+retrofit2KotlinxSerializationConverter = "1.0.0"
+appcompat = "1.6.1"
+material = "1.12.0"
+hiltNavigationCompose = "1.2.0"
+socketIoClient = "2.1.0"
+vanniktechMavenPublish = "0.28.0"
+
+[libraries]
+androidx-core-ktx = { group = "androidx.core", name = "core-ktx", version.ref = "coreKtx" }
+junit = { group = "junit", name = "junit", version.ref = "junit" }
+androidx-junit = { group = "androidx.test.ext", name = "junit", version.ref = "junitVersion" }
+androidx-espresso-core = { group = "androidx.test.espresso", name = "espresso-core", version.ref = "espressoCore" }
+androidx-lifecycle-runtime-ktx = { group = "androidx.lifecycle", name = "lifecycle-runtime-ktx", version.ref = "lifecycleRuntimeKtx" }
+androidx-activity-compose = { group = "androidx.activity", name = "activity-compose", version.ref = "activityCompose" }
+androidx-compose-bom = { group = "androidx.compose", name = "compose-bom", version.ref = "composeBom" }
+androidx-ui = { group = "androidx.compose.ui", name = "ui" }
+androidx-ui-graphics = { group = "androidx.compose.ui", name = "ui-graphics" }
+androidx-ui-tooling = { group = "androidx.compose.ui", name = "ui-tooling" }
+androidx-ui-tooling-preview = { group = "androidx.compose.ui", name = "ui-tooling-preview" }
+androidx-ui-test-manifest = { group = "androidx.compose.ui", name = "ui-test-manifest" }
+androidx-ui-test-junit4 = { group = "androidx.compose.ui", name = "ui-test-junit4" }
+androidx-material3 = { group = "androidx.compose.material3", name = "material3" }
+timber = { group = "com.jakewharton.timber", name = "timber", version.ref = "timber" }
+androidx-lifecycle-runtime-compose = { group = "androidx.lifecycle", name = "lifecycle-runtime-compose", version.ref = "lifecycleRuntimeCompose" }
+kotlinx-serialization-json = { group = "org.jetbrains.kotlinx", name = "kotlinx-serialization-json", version.ref = "kotlinx-serialization-json" }
+androidx-startup-runtime = { group = "androidx.startup", name = "startup-runtime", version.ref = "startupRuntime" }
+retrofit-bom = { group = "com.squareup.retrofit2", name = "retrofit-bom", version.ref = "retrofitBom" }
+retrofit = { group = "com.squareup.retrofit2", name = "retrofit" }
+hilt-android = { group = "com.google.dagger", name = "hilt-android", version.ref = "hilt" }
+hilt-android-compiler = { group = "com.google.dagger", name = "hilt-android-compiler", version.ref = "hilt" }
+logging-interceptor = { group = "com.squareup.okhttp3", name = "logging-interceptor", version.ref = "loggingInterceptor" }
+retrofit2-kotlinx-serialization-converter = { group = "com.jakewharton.retrofit", name = "retrofit2-kotlinx-serialization-converter", version.ref = "retrofit2KotlinxSerializationConverter" }
+androidx-appcompat = { group = "androidx.appcompat", name = "appcompat", version.ref = "appcompat" }
+material = { group = "com.google.android.material", name = "material", version.ref = "material" }
+androidx-hilt-navigation-compose = { group = "androidx.hilt", name = "hilt-navigation-compose", version.ref = "hiltNavigationCompose" }
+socket-io-client = { group = "io.socket", name = "socket.io-client", version.ref = "socketIoClient" }
+
+[plugins]
+android-application = { id = "com.android.application", version.ref = "agp" }
+jetbrains-kotlin-android = { id = "org.jetbrains.kotlin.android", version.ref = "kotlin" }
+kotlinx-serialization = { id = "org.jetbrains.kotlin.plugin.serialization", version.ref = "kotlinx-serialization-plugin" }
+hilt = { id = "com.google.dagger.hilt.android", version.ref = "hilt" }
+compose-compiler = { id = "org.jetbrains.kotlin.plugin.compose", version.ref = "kotlin" }
+ksp = { id = "com.google.devtools.ksp", version.ref = "ksp" }
+android-library = { id = "com.android.library", version.ref = "agp" }
+vanniktechMavenPublish = { id = "com.vanniktech.maven.publish", version.ref = "vanniktechMavenPublish" }
diff --git a/android/gradle/wrapper/gradle-wrapper.jar b/android/gradle/wrapper/gradle-wrapper.jar
new file mode 100644
index 0000000..e708b1c
Binary files /dev/null and b/android/gradle/wrapper/gradle-wrapper.jar differ
diff --git a/android/gradle/wrapper/gradle-wrapper.properties b/android/gradle/wrapper/gradle-wrapper.properties
new file mode 100644
index 0000000..4feaae6
--- /dev/null
+++ b/android/gradle/wrapper/gradle-wrapper.properties
@@ -0,0 +1,6 @@
+#Wed May 22 14:25:07 IST 2024
+distributionBase=GRADLE_USER_HOME
+distributionPath=wrapper/dists
+distributionUrl=https\://services.gradle.org/distributions/gradle-8.7-bin.zip
+zipStoreBase=GRADLE_USER_HOME
+zipStorePath=wrapper/dists
diff --git a/android/gradlew b/android/gradlew
new file mode 100755
index 0000000..4f906e0
--- /dev/null
+++ b/android/gradlew
@@ -0,0 +1,185 @@
+#!/usr/bin/env sh
+
+#
+# Copyright 2015 the original author or authors.
+#
+# Licensed under the Apache License, Version 2.0 (the "License");
+# you may not use this file except in compliance with the License.
+# You may obtain a copy of the License at
+#
+# https://www.apache.org/licenses/LICENSE-2.0
+#
+# Unless required by applicable law or agreed to in writing, software
+# distributed under the License is distributed on an "AS IS" BASIS,
+# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+# See the License for the specific language governing permissions and
+# limitations under the License.
+#
+
+##############################################################################
+##
+## Gradle start up script for UN*X
+##
+##############################################################################
+
+# Attempt to set APP_HOME
+# Resolve links: $0 may be a link
+PRG="$0"
+# Need this for relative symlinks.
+while [ -h "$PRG" ] ; do
+ ls=`ls -ld "$PRG"`
+ link=`expr "$ls" : '.*-> \(.*\)$'`
+ if expr "$link" : '/.*' > /dev/null; then
+ PRG="$link"
+ else
+ PRG=`dirname "$PRG"`"/$link"
+ fi
+done
+SAVED="`pwd`"
+cd "`dirname \"$PRG\"`/" >/dev/null
+APP_HOME="`pwd -P`"
+cd "$SAVED" >/dev/null
+
+APP_NAME="Gradle"
+APP_BASE_NAME=`basename "$0"`
+
+# Add default JVM options here. You can also use JAVA_OPTS and GRADLE_OPTS to pass JVM options to this script.
+DEFAULT_JVM_OPTS='"-Xmx64m" "-Xms64m"'
+
+# Use the maximum available, or set MAX_FD != -1 to use that value.
+MAX_FD="maximum"
+
+warn () {
+ echo "$*"
+}
+
+die () {
+ echo
+ echo "$*"
+ echo
+ exit 1
+}
+
+# OS specific support (must be 'true' or 'false').
+cygwin=false
+msys=false
+darwin=false
+nonstop=false
+case "`uname`" in
+ CYGWIN* )
+ cygwin=true
+ ;;
+ Darwin* )
+ darwin=true
+ ;;
+ MINGW* )
+ msys=true
+ ;;
+ NONSTOP* )
+ nonstop=true
+ ;;
+esac
+
+CLASSPATH=$APP_HOME/gradle/wrapper/gradle-wrapper.jar
+
+
+# Determine the Java command to use to start the JVM.
+if [ -n "$JAVA_HOME" ] ; then
+ if [ -x "$JAVA_HOME/jre/sh/java" ] ; then
+ # IBM's JDK on AIX uses strange locations for the executables
+ JAVACMD="$JAVA_HOME/jre/sh/java"
+ else
+ JAVACMD="$JAVA_HOME/bin/java"
+ fi
+ if [ ! -x "$JAVACMD" ] ; then
+ die "ERROR: JAVA_HOME is set to an invalid directory: $JAVA_HOME
+
+Please set the JAVA_HOME variable in your environment to match the
+location of your Java installation."
+ fi
+else
+ JAVACMD="java"
+ which java >/dev/null 2>&1 || die "ERROR: JAVA_HOME is not set and no 'java' command could be found in your PATH.
+
+Please set the JAVA_HOME variable in your environment to match the
+location of your Java installation."
+fi
+
+# Increase the maximum file descriptors if we can.
+if [ "$cygwin" = "false" -a "$darwin" = "false" -a "$nonstop" = "false" ] ; then
+ MAX_FD_LIMIT=`ulimit -H -n`
+ if [ $? -eq 0 ] ; then
+ if [ "$MAX_FD" = "maximum" -o "$MAX_FD" = "max" ] ; then
+ MAX_FD="$MAX_FD_LIMIT"
+ fi
+ ulimit -n $MAX_FD
+ if [ $? -ne 0 ] ; then
+ warn "Could not set maximum file descriptor limit: $MAX_FD"
+ fi
+ else
+ warn "Could not query maximum file descriptor limit: $MAX_FD_LIMIT"
+ fi
+fi
+
+# For Darwin, add options to specify how the application appears in the dock
+if $darwin; then
+ GRADLE_OPTS="$GRADLE_OPTS \"-Xdock:name=$APP_NAME\" \"-Xdock:icon=$APP_HOME/media/gradle.icns\""
+fi
+
+# For Cygwin or MSYS, switch paths to Windows format before running java
+if [ "$cygwin" = "true" -o "$msys" = "true" ] ; then
+ APP_HOME=`cygpath --path --mixed "$APP_HOME"`
+ CLASSPATH=`cygpath --path --mixed "$CLASSPATH"`
+
+ JAVACMD=`cygpath --unix "$JAVACMD"`
+
+ # We build the pattern for arguments to be converted via cygpath
+ ROOTDIRSRAW=`find -L / -maxdepth 1 -mindepth 1 -type d 2>/dev/null`
+ SEP=""
+ for dir in $ROOTDIRSRAW ; do
+ ROOTDIRS="$ROOTDIRS$SEP$dir"
+ SEP="|"
+ done
+ OURCYGPATTERN="(^($ROOTDIRS))"
+ # Add a user-defined pattern to the cygpath arguments
+ if [ "$GRADLE_CYGPATTERN" != "" ] ; then
+ OURCYGPATTERN="$OURCYGPATTERN|($GRADLE_CYGPATTERN)"
+ fi
+ # Now convert the arguments - kludge to limit ourselves to /bin/sh
+ i=0
+ for arg in "$@" ; do
+ CHECK=`echo "$arg"|egrep -c "$OURCYGPATTERN" -`
+ CHECK2=`echo "$arg"|egrep -c "^-"` ### Determine if an option
+
+ if [ $CHECK -ne 0 ] && [ $CHECK2 -eq 0 ] ; then ### Added a condition
+ eval `echo args$i`=`cygpath --path --ignore --mixed "$arg"`
+ else
+ eval `echo args$i`="\"$arg\""
+ fi
+ i=`expr $i + 1`
+ done
+ case $i in
+ 0) set -- ;;
+ 1) set -- "$args0" ;;
+ 2) set -- "$args0" "$args1" ;;
+ 3) set -- "$args0" "$args1" "$args2" ;;
+ 4) set -- "$args0" "$args1" "$args2" "$args3" ;;
+ 5) set -- "$args0" "$args1" "$args2" "$args3" "$args4" ;;
+ 6) set -- "$args0" "$args1" "$args2" "$args3" "$args4" "$args5" ;;
+ 7) set -- "$args0" "$args1" "$args2" "$args3" "$args4" "$args5" "$args6" ;;
+ 8) set -- "$args0" "$args1" "$args2" "$args3" "$args4" "$args5" "$args6" "$args7" ;;
+ 9) set -- "$args0" "$args1" "$args2" "$args3" "$args4" "$args5" "$args6" "$args7" "$args8" ;;
+ esac
+fi
+
+# Escape application args
+save () {
+ for i do printf %s\\n "$i" | sed "s/'/'\\\\''/g;1s/^/'/;\$s/\$/' \\\\/" ; done
+ echo " "
+}
+APP_ARGS=`save "$@"`
+
+# Collect all arguments for the java command, following the shell quoting and substitution rules
+eval set -- $DEFAULT_JVM_OPTS $JAVA_OPTS $GRADLE_OPTS "\"-Dorg.gradle.appname=$APP_BASE_NAME\"" -classpath "\"$CLASSPATH\"" org.gradle.wrapper.GradleWrapperMain "$APP_ARGS"
+
+exec "$JAVACMD" "$@"
diff --git a/android/gradlew.bat b/android/gradlew.bat
new file mode 100644
index 0000000..107acd3
--- /dev/null
+++ b/android/gradlew.bat
@@ -0,0 +1,89 @@
+@rem
+@rem Copyright 2015 the original author or authors.
+@rem
+@rem Licensed under the Apache License, Version 2.0 (the "License");
+@rem you may not use this file except in compliance with the License.
+@rem You may obtain a copy of the License at
+@rem
+@rem https://www.apache.org/licenses/LICENSE-2.0
+@rem
+@rem Unless required by applicable law or agreed to in writing, software
+@rem distributed under the License is distributed on an "AS IS" BASIS,
+@rem WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+@rem See the License for the specific language governing permissions and
+@rem limitations under the License.
+@rem
+
+@if "%DEBUG%" == "" @echo off
+@rem ##########################################################################
+@rem
+@rem Gradle startup script for Windows
+@rem
+@rem ##########################################################################
+
+@rem Set local scope for the variables with windows NT shell
+if "%OS%"=="Windows_NT" setlocal
+
+set DIRNAME=%~dp0
+if "%DIRNAME%" == "" set DIRNAME=.
+set APP_BASE_NAME=%~n0
+set APP_HOME=%DIRNAME%
+
+@rem Resolve any "." and ".." in APP_HOME to make it shorter.
+for %%i in ("%APP_HOME%") do set APP_HOME=%%~fi
+
+@rem Add default JVM options here. You can also use JAVA_OPTS and GRADLE_OPTS to pass JVM options to this script.
+set DEFAULT_JVM_OPTS="-Xmx64m" "-Xms64m"
+
+@rem Find java.exe
+if defined JAVA_HOME goto findJavaFromJavaHome
+
+set JAVA_EXE=java.exe
+%JAVA_EXE% -version >NUL 2>&1
+if "%ERRORLEVEL%" == "0" goto execute
+
+echo.
+echo ERROR: JAVA_HOME is not set and no 'java' command could be found in your PATH.
+echo.
+echo Please set the JAVA_HOME variable in your environment to match the
+echo location of your Java installation.
+
+goto fail
+
+:findJavaFromJavaHome
+set JAVA_HOME=%JAVA_HOME:"=%
+set JAVA_EXE=%JAVA_HOME%/bin/java.exe
+
+if exist "%JAVA_EXE%" goto execute
+
+echo.
+echo ERROR: JAVA_HOME is set to an invalid directory: %JAVA_HOME%
+echo.
+echo Please set the JAVA_HOME variable in your environment to match the
+echo location of your Java installation.
+
+goto fail
+
+:execute
+@rem Setup the command line
+
+set CLASSPATH=%APP_HOME%\gradle\wrapper\gradle-wrapper.jar
+
+
+@rem Execute Gradle
+"%JAVA_EXE%" %DEFAULT_JVM_OPTS% %JAVA_OPTS% %GRADLE_OPTS% "-Dorg.gradle.appname=%APP_BASE_NAME%" -classpath "%CLASSPATH%" org.gradle.wrapper.GradleWrapperMain %*
+
+:end
+@rem End local scope for the variables with windows NT shell
+if "%ERRORLEVEL%"=="0" goto mainEnd
+
+:fail
+rem Set variable GRADLE_EXIT_CONSOLE if you need the _script_ return code instead of
+rem the _cmd.exe /c_ return code!
+if not "" == "%GRADLE_EXIT_CONSOLE%" exit 1
+exit /b 1
+
+:mainEnd
+if "%OS%"=="Windows_NT" endlocal
+
+:omega
diff --git a/android/settings.gradle.kts b/android/settings.gradle.kts
new file mode 100644
index 0000000..0668f97
--- /dev/null
+++ b/android/settings.gradle.kts
@@ -0,0 +1,26 @@
+pluginManagement {
+ repositories {
+ google {
+ content {
+ includeGroupByRegex("com\\.android.*")
+ includeGroupByRegex("com\\.google.*")
+ includeGroupByRegex("androidx.*")
+ }
+ }
+ mavenLocal()
+ mavenCentral()
+ gradlePluginPortal()
+ }
+}
+dependencyResolutionManagement {
+ repositoriesMode.set(RepositoriesMode.FAIL_ON_PROJECT_REPOS)
+ repositories {
+ google()
+ mavenLocal()
+ mavenCentral()
+ }
+}
+
+rootProject.name = "BagelAndroid"
+include(":app")
+include(":bagel")