diff --git a/.github/workflows/test.yml b/.github/workflows/test.yml
new file mode 100644
index 0000000..c653388
--- /dev/null
+++ b/.github/workflows/test.yml
@@ -0,0 +1,54 @@
+name: Test
+on:
+ push:
+ branches: ['master']
+ pull_request:
+
+jobs:
+ test:
+ runs-on: ubuntu-latest
+
+ env:
+ RAILS_ENV: test
+
+ strategy:
+ fail-fast: false
+ matrix:
+ include:
+ ### TEST RUBY VERSIONS
+ - ruby: "2.5"
+ - ruby: "2.6"
+ - ruby: "2.7"
+ - ruby: "3.0"
+ - ruby: "3.1"
+ - ruby: "3.2"
+ ### TEST RAILS VERSIONS
+ - ruby: "2.6"
+ env:
+ RAILS_VERSION: "5.2"
+ - ruby: "2.6"
+ env:
+ RAILS_VERSION: "6.0"
+ - ruby: "2.6"
+ env:
+ RAILS_VERSION: "6.1"
+ - ruby: "3.2"
+ env:
+ RAILS_VERSION: "7.0"
+ - ruby: "3.2"
+ env:
+ RAILS_VERSION: "7.1"
+
+ steps:
+ - uses: actions/checkout@v3
+
+ - name: Install ruby
+ uses: ruby/setup-ruby@v1
+ with:
+ ruby-version: "${{ matrix.ruby }}"
+ bundler-cache: false ### not compatible with ENV style gemfile
+
+ - name: Run test
+ run: |
+ bundle install
+ bundle exec rake test
diff --git a/.gitignore b/.gitignore
index 0534de3..7933607 100644
--- a/.gitignore
+++ b/.gitignore
@@ -10,3 +10,6 @@
/test/*.sqlite3*
/production/*.sqlite3*
.DS_Store
+/test/dummy_app/**/*.sqlite3*
+/test/dummy_app/**/*.log
+/test/dummy_app/tmp/
diff --git a/CHANGELOG.md b/CHANGELOG.md
index 10e0969..e09b201 100644
--- a/CHANGELOG.md
+++ b/CHANGELOG.md
@@ -5,6 +5,7 @@
- Fix Litesearch tests
- Suppress chatty Litejob exit detector when there are no jobs in flight
- Tidy up the test folder
+- [#xx](https://github.com/oldmoe/litestack/pull/xx) - Add dummy Rails app for test suite and Github CI workflow
## [0.4.1] - 2023-10-11
diff --git a/Gemfile b/Gemfile
index e556cfc..3bbc88a 100644
--- a/Gemfile
+++ b/Gemfile
@@ -4,3 +4,9 @@ source "https://rubygems.org"
# Specify your gem's dependencies in litestack.gemspec
gemspec
+
+def get_env(name)
+ (ENV[name] && !ENV[name].empty?) ? ENV[name] : nil
+end
+
+gem 'rails', get_env("RAILS_VERSION")
diff --git a/README.md b/README.md
index ea65317..af9c658 100644
--- a/README.md
+++ b/README.md
@@ -1,5 +1,7 @@
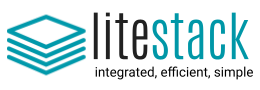
+
+
All your data infrastructure, in a gem!
Litestack is a Ruby gem that provides both Ruby and Ruby on Rails applications an all-in-one solution for web application data infrastructure. It exploits the power and embeddedness of SQLite to deliver a full-fledged SQL database, a fast cache , a robust job queue, a reliable message broker, a full text search engine and a metrics platform all in a single package.
diff --git a/test/dummy_app/Rakefile b/test/dummy_app/Rakefile
new file mode 100644
index 0000000..3645852
--- /dev/null
+++ b/test/dummy_app/Rakefile
@@ -0,0 +1,7 @@
+#!/usr/bin/env rake
+# Add your own tasks in files placed in lib/tasks ending in .rake,
+# for example lib/tasks/capistrano.rake, and they will automatically be available to Rake.
+
+require File.expand_path('../config/application', __FILE__)
+
+Dummy::Application.load_tasks
diff --git a/test/dummy_app/app/assets/config/manifest.js b/test/dummy_app/app/assets/config/manifest.js
new file mode 100644
index 0000000..b9fe2b7
--- /dev/null
+++ b/test/dummy_app/app/assets/config/manifest.js
@@ -0,0 +1,3 @@
+//= link_tree ../images
+//= link_directory ../javascripts .js
+//= link_directory ../stylesheets .css .scss
diff --git a/test/dummy_app/app/assets/javascripts/application.js b/test/dummy_app/app/assets/javascripts/application.js
new file mode 100644
index 0000000..e69de29
diff --git a/test/dummy_app/app/assets/stylesheets/application.css b/test/dummy_app/app/assets/stylesheets/application.css
new file mode 100644
index 0000000..371848b
--- /dev/null
+++ b/test/dummy_app/app/assets/stylesheets/application.css
@@ -0,0 +1,3 @@
+/*
+ *= require_self
+*/
diff --git a/test/dummy_app/app/channels/application_cable.rb b/test/dummy_app/app/channels/application_cable.rb
new file mode 100644
index 0000000..5c28f8d
--- /dev/null
+++ b/test/dummy_app/app/channels/application_cable.rb
@@ -0,0 +1,7 @@
+module ApplicationCable
+ class Channel < ActionCable::Channel::Base
+ end
+
+ class Connection < ActionCable::Connection::Base
+ end
+end
diff --git a/test/dummy_app/app/controllers/application_controller.rb b/test/dummy_app/app/controllers/application_controller.rb
new file mode 100644
index 0000000..e8065d9
--- /dev/null
+++ b/test/dummy_app/app/controllers/application_controller.rb
@@ -0,0 +1,3 @@
+class ApplicationController < ActionController::Base
+ protect_from_forgery
+end
diff --git a/test/dummy_app/app/jobs/application_job.rb b/test/dummy_app/app/jobs/application_job.rb
new file mode 100644
index 0000000..a009ace
--- /dev/null
+++ b/test/dummy_app/app/jobs/application_job.rb
@@ -0,0 +1,2 @@
+class ApplicationJob < ActiveJob::Base
+end
diff --git a/test/dummy_app/app/mailers/.gitkeep b/test/dummy_app/app/mailers/.gitkeep
new file mode 100644
index 0000000..e69de29
diff --git a/test/dummy_app/app/models/application_record.rb b/test/dummy_app/app/models/application_record.rb
new file mode 100644
index 0000000..10a4cba
--- /dev/null
+++ b/test/dummy_app/app/models/application_record.rb
@@ -0,0 +1,3 @@
+class ApplicationRecord < ActiveRecord::Base
+ self.abstract_class = true
+end
diff --git a/test/dummy_app/app/models/comment.rb b/test/dummy_app/app/models/comment.rb
new file mode 100644
index 0000000..8b86c56
--- /dev/null
+++ b/test/dummy_app/app/models/comment.rb
@@ -0,0 +1,3 @@
+class Comment < ApplicationRecord
+ belongs_to :post
+end
diff --git a/test/dummy_app/app/models/post.rb b/test/dummy_app/app/models/post.rb
new file mode 100644
index 0000000..4a0df21
--- /dev/null
+++ b/test/dummy_app/app/models/post.rb
@@ -0,0 +1,3 @@
+class Post < ApplicationRecord
+ has_many :comments
+end
diff --git a/test/dummy_app/app/views/layouts/application.html.erb b/test/dummy_app/app/views/layouts/application.html.erb
new file mode 100644
index 0000000..be7e6dc
--- /dev/null
+++ b/test/dummy_app/app/views/layouts/application.html.erb
@@ -0,0 +1,14 @@
+
+
+
+ Dummy App
+ <%= stylesheet_link_tag "application" %>
+ <%= javascript_include_tag "application" %>
+ <%= csrf_meta_tags %>
+
+
+
+<%= yield %>
+
+
+
diff --git a/test/dummy_app/config.ru b/test/dummy_app/config.ru
new file mode 100644
index 0000000..1989ed8
--- /dev/null
+++ b/test/dummy_app/config.ru
@@ -0,0 +1,4 @@
+# This file is used by Rack-based servers to start the application.
+
+require ::File.expand_path('../config/environment', __FILE__)
+run Dummy::Application
diff --git a/test/dummy_app/config/application.rb b/test/dummy_app/config/application.rb
new file mode 100644
index 0000000..f53f525
--- /dev/null
+++ b/test/dummy_app/config/application.rb
@@ -0,0 +1,48 @@
+require File.expand_path('../boot', __FILE__)
+
+require 'rails/all'
+
+Bundler.require
+
+module Dummy
+ class Application < Rails::Application
+ config.load_defaults Rails::VERSION::STRING.to_f
+
+ # Settings in config/environments/* take precedence over those specified here.
+ # Application configuration should go into files in config/initializers
+ # -- all .rb files in that directory are automatically loaded.
+
+ # Custom directories with classes and modules you want to be autoloadable.
+ # config.autoload_paths += %W(#{config.root}/extras)
+
+ # Only load the plugins named here, in the order given (default is alphabetical).
+ # :all can be used as a placeholder for all plugins not explicitly named.
+ # config.plugins = [ :exception_notification, :ssl_requirement, :all ]
+
+ # Activate observers that should always be running.
+ # config.active_record.observers = :cacher, :garbage_collector, :forum_observer
+
+ # Set Time.zone default to the specified zone and make Active Record auto-convert to this zone.
+ # Run "rake -D time" for a list of tasks for finding time zone names. Default is UTC.
+ # config.time_zone = 'Central Time (US & Canada)'
+
+ # The default locale is :en and all translations from config/locales/*.rb,yml are auto loaded.
+ # config.i18n.load_path += Dir[Rails.root.join('my', 'locales', '*.{rb,yml}').to_s]
+ # config.i18n.default_locale = :de
+
+ # Configure the default encoding used in templates for Ruby 1.9.
+ config.encoding = "utf-8"
+
+ # Configure sensitive parameters which will be filtered from the log file.
+ config.filter_parameters += [:password]
+
+ config.generators.test_framework = false
+ config.generators.helper = false
+ config.generators.stylesheets = false
+ config.generators.javascripts = false
+
+ config.after_initialize do
+ ActiveRecord::Migration.migrate(Rails.root.join("db/migrate/*").to_s)
+ end
+ end
+end
diff --git a/test/dummy_app/config/boot.rb b/test/dummy_app/config/boot.rb
new file mode 100644
index 0000000..eba0681
--- /dev/null
+++ b/test/dummy_app/config/boot.rb
@@ -0,0 +1,10 @@
+require 'rubygems'
+gemfile = File.expand_path('../../../../Gemfile', __FILE__)
+
+if File.exist?(gemfile)
+ ENV['BUNDLE_GEMFILE'] = gemfile
+ require 'bundler'
+ Bundler.setup
+end
+
+$:.unshift File.expand_path('../../../../lib', __FILE__)
\ No newline at end of file
diff --git a/test/dummy_app/config/cable.yml b/test/dummy_app/config/cable.yml
new file mode 100644
index 0000000..3425c73
--- /dev/null
+++ b/test/dummy_app/config/cable.yml
@@ -0,0 +1,6 @@
+development:
+ adapter: litecable
+
+test:
+ adapter: test
+ #adapter: litecable
diff --git a/test/dummy_app/config/database.yml b/test/dummy_app/config/database.yml
new file mode 100644
index 0000000..9b7dc26
--- /dev/null
+++ b/test/dummy_app/config/database.yml
@@ -0,0 +1,7 @@
+development:
+ adapter: litedb
+ database: db/development/database.sqlite3.db
+
+test:
+ adapter: litedb
+ database: db/test/database.sqlite3.db
diff --git a/test/dummy_app/config/environment.rb b/test/dummy_app/config/environment.rb
new file mode 100644
index 0000000..3da5eb9
--- /dev/null
+++ b/test/dummy_app/config/environment.rb
@@ -0,0 +1,5 @@
+# Load the rails application
+require File.expand_path('../application', __FILE__)
+
+# Initialize the rails application
+Dummy::Application.initialize!
diff --git a/test/dummy_app/config/environments/test.rb b/test/dummy_app/config/environments/test.rb
new file mode 100644
index 0000000..225dd97
--- /dev/null
+++ b/test/dummy_app/config/environments/test.rb
@@ -0,0 +1,41 @@
+Dummy::Application.configure do
+ # Settings specified here will take precedence over those in config/application.rb
+
+ # The test environment is used exclusively to run your application's
+ # test suite. You never need to work with it otherwise. Remember that
+ # your test database is "scratch space" for the test suite and is wiped
+ # and recreated between test runs. Don't rely on the data there!
+ config.cache_classes = true
+
+ # Configure static asset server for tests with Cache-Control for performance
+ config.serve_static_assets = true
+ config.public_file_server.headers = { 'Cache-Control' => 'public, max-age=3600' }
+
+ # Log error messages when you accidentally call methods on nil
+ config.whiny_nils = true
+
+ # Show full error reports and disable caching
+ config.consider_all_requests_local = true
+ config.action_controller.perform_caching = false
+
+ # Raise exceptions instead of rendering exception templates
+ config.action_dispatch.show_exceptions = false
+
+ # Disable request forgery protection in test environment
+ config.action_controller.allow_forgery_protection = false
+
+ # Tell Action Mailer not to deliver emails to the real world.
+ # The :test delivery method accumulates sent emails in the
+ # ActionMailer::Base.deliveries array.
+ config.action_mailer.delivery_method = :test
+
+ # Use SQL instead of Active Record's schema dumper when creating the test database.
+ # This is necessary if your schema can't be completely dumped by the schema dumper,
+ # like if you have constraints or database-specific column types
+ # config.active_record.schema_format = :sql
+
+ # Print deprecation notices to the stderr
+ config.active_support.deprecation = :stderr
+
+ config.eager_load = false
+end
diff --git a/test/dummy_app/config/initializers/backtrace_silencers.rb b/test/dummy_app/config/initializers/backtrace_silencers.rb
new file mode 100644
index 0000000..59385cd
--- /dev/null
+++ b/test/dummy_app/config/initializers/backtrace_silencers.rb
@@ -0,0 +1,7 @@
+# Be sure to restart your server when you modify this file.
+
+# You can add backtrace silencers for libraries that you're using but don't wish to see in your backtraces.
+# Rails.backtrace_cleaner.add_silencer { |line| line =~ /my_noisy_library/ }
+
+# You can also remove all the silencers if you're trying to debug a problem that might stem from framework code.
+# Rails.backtrace_cleaner.remove_silencers!
diff --git a/test/dummy_app/config/initializers/inflections.rb b/test/dummy_app/config/initializers/inflections.rb
new file mode 100644
index 0000000..9e8b013
--- /dev/null
+++ b/test/dummy_app/config/initializers/inflections.rb
@@ -0,0 +1,10 @@
+# Be sure to restart your server when you modify this file.
+
+# Add new inflection rules using the following format
+# (all these examples are active by default):
+# ActiveSupport::Inflector.inflections do |inflect|
+# inflect.plural /^(ox)$/i, '\1en'
+# inflect.singular /^(ox)en/i, '\1'
+# inflect.irregular 'person', 'people'
+# inflect.uncountable %w( fish sheep )
+# end
diff --git a/test/dummy_app/config/initializers/mime_types.rb b/test/dummy_app/config/initializers/mime_types.rb
new file mode 100644
index 0000000..72aca7e
--- /dev/null
+++ b/test/dummy_app/config/initializers/mime_types.rb
@@ -0,0 +1,5 @@
+# Be sure to restart your server when you modify this file.
+
+# Add new mime types for use in respond_to blocks:
+# Mime::Type.register "text/richtext", :rtf
+# Mime::Type.register_alias "text/html", :iphone
diff --git a/test/dummy_app/config/initializers/secret_token.rb b/test/dummy_app/config/initializers/secret_token.rb
new file mode 100644
index 0000000..4e00665
--- /dev/null
+++ b/test/dummy_app/config/initializers/secret_token.rb
@@ -0,0 +1,11 @@
+# Be sure to restart your server when you modify this file.
+
+# Your secret key for verifying the integrity of signed cookies.
+# If you change this key, all old signed cookies will become invalid!
+# Make sure the secret is at least 30 characters and all random,
+# no regular words or you'll be exposed to dictionary attacks.
+
+gem_version = ActiveRecord.gem_version
+if gem_version <= Gem::Version.new("5.1")
+ Dummy::Application.config.secret_token = '4f337f0063fbb4a724dd8da15419679300da990ae4f6c94d36c714a3cd07e9653fc42d902cf33a9b9449a28e7eb2673f928172d65a090fa3c9156d6beea8d16c'
+end
diff --git a/test/dummy_app/config/initializers/session_store.rb b/test/dummy_app/config/initializers/session_store.rb
new file mode 100644
index 0000000..952473f
--- /dev/null
+++ b/test/dummy_app/config/initializers/session_store.rb
@@ -0,0 +1,8 @@
+# Be sure to restart your server when you modify this file.
+
+Dummy::Application.config.session_store :cookie_store, key: '_dummy_session'
+
+# Use the database for sessions instead of the cookie-based default,
+# which shouldn't be used to store highly confidential information
+# (create the session table with "rails generate session_migration")
+# Dummy::Application.config.session_store :active_record_store
diff --git a/test/dummy_app/config/initializers/wrap_parameters.rb b/test/dummy_app/config/initializers/wrap_parameters.rb
new file mode 100644
index 0000000..999df20
--- /dev/null
+++ b/test/dummy_app/config/initializers/wrap_parameters.rb
@@ -0,0 +1,14 @@
+# Be sure to restart your server when you modify this file.
+#
+# This file contains settings for ActionController::ParamsWrapper which
+# is enabled by default.
+
+# Enable parameter wrapping for JSON. You can disable this by setting :format to an empty array.
+ActiveSupport.on_load(:action_controller) do
+ wrap_parameters format: [:json]
+end
+
+# Disable root element in JSON by default.
+ActiveSupport.on_load(:active_record) do
+ self.include_root_in_json = false
+end
diff --git a/test/dummy_app/config/locales/en.yml b/test/dummy_app/config/locales/en.yml
new file mode 100644
index 0000000..179c14c
--- /dev/null
+++ b/test/dummy_app/config/locales/en.yml
@@ -0,0 +1,5 @@
+# Sample localization file for English. Add more files in this directory for other locales.
+# See https://github.com/svenfuchs/rails-i18n/tree/master/rails%2Flocale for starting points.
+
+en:
+ hello: "Hello world"
diff --git a/test/dummy_app/config/routes.rb b/test/dummy_app/config/routes.rb
new file mode 100644
index 0000000..b7b20d0
--- /dev/null
+++ b/test/dummy_app/config/routes.rb
@@ -0,0 +1,2 @@
+Dummy::Application.routes.draw do
+end
diff --git a/test/dummy_app/config/secrets.yml b/test/dummy_app/config/secrets.yml
new file mode 100644
index 0000000..1238a0c
--- /dev/null
+++ b/test/dummy_app/config/secrets.yml
@@ -0,0 +1,22 @@
+# Be sure to restart your server when you modify this file.
+
+# Your secret key is used for verifying the integrity of signed cookies.
+# If you change this key, all old signed cookies will become invalid!
+
+# Make sure the secret is at least 30 characters and all random,
+# no regular words or you'll be exposed to dictionary attacks.
+# You can use `rake secret` to generate a secure secret key.
+
+# Make sure the secrets in this file are kept private
+# if you're sharing your code publicly.
+
+development:
+ secret_key_base: d28054e102cd55dcd684cee239d31ddf1e1acd83bd879dd5f671e989f5c9d94ec1ede00e7fcf9b6bde4cd115f93c54e3ba6c5dc05d233292542f27a79706fcb4
+
+test:
+ secret_key_base: 378b4f2309d4898f5170b41624e19bf60ce8a154ad87c100e8846bddcf4c28b72b533f2e73738ef8f6eabb7a773a0a0e7c32c0649916c5f280eb7ac621fc318c
+
+# Do not keep production secrets in the repository,
+# instead read values from the environment.
+production:
+ secret_key_base: 5e73c057b92f67f980fbea4c1c2c495b25def0048f8c1c040fed9c08f49cd50a2ebf872dd87857afc0861479e9382fceb7d9837a0bce546c2f7594e2f4da45e3
diff --git a/test/dummy_app/db/migrate/20231102155312_setup_test_tables.rb b/test/dummy_app/db/migrate/20231102155312_setup_test_tables.rb
new file mode 100644
index 0000000..4cb2c0d
--- /dev/null
+++ b/test/dummy_app/db/migrate/20231102155312_setup_test_tables.rb
@@ -0,0 +1,16 @@
+class SetupTestTables < ActiveRecord::Migration::Current
+
+ def change
+ create_table :posts do |t|
+ t.text :title, :content
+ t.timestamps
+ end
+
+ create_table :comments do |t|
+ t.string :content
+ t.references :post
+ t.timestamps
+ end
+ end
+
+end
diff --git a/test/dummy_app/log/.gitkeep b/test/dummy_app/log/.gitkeep
new file mode 100644
index 0000000..e69de29
diff --git a/test/models/.gitkeep b/test/models/.gitkeep
new file mode 100644
index 0000000..e69de29
diff --git a/test/rails_helper.rb b/test/rails_helper.rb
new file mode 100644
index 0000000..595d10b
--- /dev/null
+++ b/test/rails_helper.rb
@@ -0,0 +1,30 @@
+ENV["RAILS_ENV"] = "test"
+
+require "active_support/all"
+
+### Delete the database completely before starting
+FileUtils.rm(
+ File.expand_path("../dummy_app/db/tests/*sqlite*", __FILE__),
+ force: true,
+)
+
+### Instantiates Rails
+require File.expand_path("../dummy_app/config/environment.rb", __FILE__)
+
+require "rails/test_help"
+
+class ActiveSupport::TestCase
+ # Setup all fixtures in test/fixtures/*.yml for all tests in alphabetical order.
+ fixtures :all
+end
+
+Rails.backtrace_cleaner.remove_silencers!
+
+require "minitest/autorun"
+
+# Run any available migration
+if ActiveRecord.gem_version >= Gem::Version.new("6.0")
+ ActiveRecord::MigrationContext.new(File.expand_path("dummy_app/db/migrate/", __dir__), ActiveRecord::SchemaMigration).migrate
+else
+ ActiveRecord::MigrationContext.new(File.expand_path("dummy_app/db/migrate/", __dir__)).migrate
+end
diff --git a/test/request/.gitkeep b/test/request/.gitkeep
new file mode 100644
index 0000000..e69de29
diff --git a/test/system/.gitkeep b/test/system/.gitkeep
new file mode 100644
index 0000000..e69de29
diff --git a/test/test_helper.rb b/test/test_helper.rb
index 54b1d6b..5128b1a 100644
--- a/test/test_helper.rb
+++ b/test/test_helper.rb
@@ -8,6 +8,8 @@
enable_coverage :branch
end
+require_relative "rails_helper"
+
require "litestack"
require "minitest/autorun"